JavaScript: Retrieve the value of a specified property from all elements in an array
JavaScript Array: Exercise-27 with Solution
Retrieve Property Values
Write a JavaScript function to retrieve the value of a given property from all elements in an array.
Sample array: [NaN, 0, 15, false, -22, '',undefined, 47, null]
Expected result:
[15, -22, 47]
Sample Solution:
JavaScript Code:
// Array of book objects in the library
var library = [
{ author: 'Bill Gates', title: 'The Road Ahead', libraryID: 1254},
{ author: 'Steve Jobs', title: 'Walter Isaacson', libraryID: 4264},
{ author: 'Suzanne Collins', title: 'Mockingjay: The Final Book of The Hunger Games', libraryID: 3245}
];
// Function to extract values of a specified property from objects in an array
function property_value(array, property_key) {
// Initialize an empty array 'arr'
var arr = [],
// Initialize index to -1, arrlen to the length of the array (if not provided, default to 0),
// array_items to store the current array item
index = -1,
arrlen = array.length,
array_items;
// Iterate through the elements of the array using a while loop
while (++index < arrlen) {
// Get the current array item
array_items = array[index];
// Check if the array item has the specified property
if (array_items.hasOwnProperty(property_key)) {
// If the property exists, add its value to the 'arr' array
arr[arr.length] = array_items[property_key];
}
}
// Return the 'arr' array containing values of the specified property
return arr;
}
// Output the result of the property_value function with the 'title' property
console.log(property_value(library, 'title'));
// Output the result of the property_value function with the 'author' property
console.log(property_value(library, 'author'));
Output:
["The Road Ahead","Walter Isaacson","Mockingjay: The Final Book of The Hunger Games"] ["Bill Gates","Steve Jobs","Suzanne Collins"]
Flowchart:
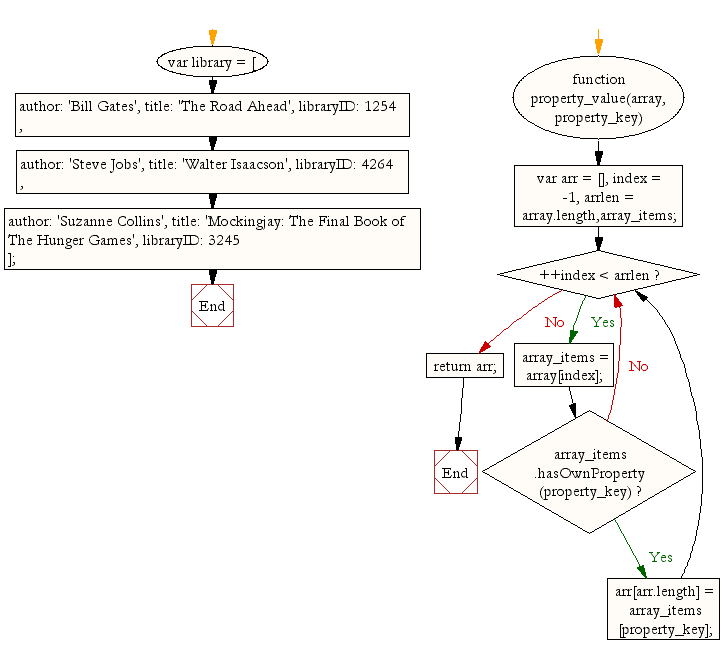
ES6 Version:
// Array of book objects in the library
const library = [
{ author: 'Bill Gates', title: 'The Road Ahead', libraryID: 1254},
{ author: 'Steve Jobs', title: 'Walter Isaacson', libraryID: 4264},
{ author: 'Suzanne Collins', title: 'Mockingjay: The Final Book of The Hunger Games', libraryID: 3245}
];
// Function to extract values of a specified property from objects in an array
const property_value = (array, property_key) => {
// Initialize an empty array 'arr'
const arr = [];
// Iterate through the elements of the array using a for...of loop
for (const array_items of array) {
// Check if the array item has the specified property
if (array_items.hasOwnProperty(property_key)) {
// If the property exists, add its value to the 'arr' array
arr.push(array_items[property_key]);
}
}
// Return the 'arr' array containing values of the specified property
return arr;
};
// Output the result of the property_value function with the 'title' property
console.log(property_value(library, 'title'));
// Output the result of the property_value function with the 'author' property
console.log(property_value(library, 'author'));
Live Demo:
See the Pen JavaScript - Retrieve the value of a specified property from all elements in an array - array-ex- 27 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program to find a pair of elements (indices of the two numbers) from an given array whose sum equals a specific target number.
Next: Write a JavaScript function to find the longest common starting substring in a set of strings.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics