JavaScript: Find a pair of elements from an specified array whose sum equals a specific target number
JavaScript Array: Exercise-26 with Solution
Find Pair with Target Sum
Write a JavaScript program to find a pair of elements (indices of the two numbers) in a given array whose sum equals a specific target number.
Input: numbers= [10,20,10,40,50,60,70], target=50
Output: 2, 3
Visual Presentation:
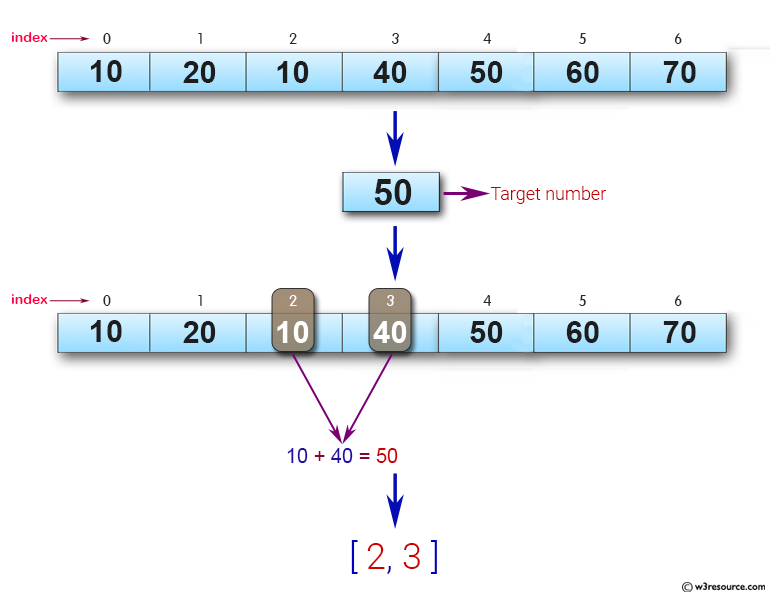
Sample Solution:
JavaScript Code:
// Function to find two indices in the array 'nums' whose elements sum to the 'target_num'
function twoSum(nums, target_num) {
// Initialize an empty array 'map' to store the difference between 'target_num' and elements in 'nums'
var map = [];
// Initialize an empty array 'indexnum' to store the indices of the two numbers
var indexnum = [];
// Iterate through each element in the 'nums' array
for (var x = 0; x < nums.length; x++) {
// Check if the current element's complement exists in the 'map' array
if (map[nums[x]] != null) {
// If found, store the indices of the two numbers and break out of the loop
var index = map[nums[x]];
indexnum[0] = index;
indexnum[1] = x;
break;
} else {
// If not found, store the index of the current element's complement in the 'map' array
map[target_num - nums[x]] = x;
}
}
// Return the array containing the indices of the two numbers
return indexnum;
}
// Output the result of the twoSum function with a sample array and target number
console.log(twoSum([10,20,10,40,50,60,70], 50));
Output:
[2,3]
Flowchart:
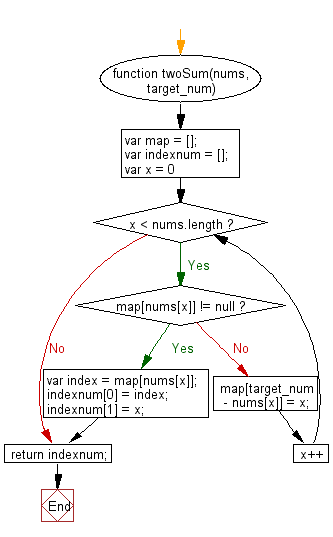
ES6 Version:
// Function to find two indices in the array 'nums' whose elements sum to the 'target_num'
const twoSum = (nums, target_num) => {
// Initialize an empty array 'map' to store the difference between 'target_num' and elements in 'nums'
const map = [];
// Initialize an empty array 'indexnum' to store the indices of the two numbers
const indexnum = [];
// Iterate through each element in the 'nums' array
for (let x = 0; x < nums.length; x++) {
// Check if the current element's complement exists in the 'map' array
if (map[nums[x]] != null) {
// If found, store the indices of the two numbers and break out of the loop
const index = map[nums[x]];
indexnum[0] = index;
indexnum[1] = x;
break;
} else {
// If not found, store the index of the current element's complement in the 'map' array
map[target_num - nums[x]] = x;
}
}
// Return the array containing the indices of the two numbers
return indexnum;
};
// Output the result of the twoSum function with a sample array and target number
console.log(twoSum([10, 20, 10, 40, 50, 60, 70], 50));
Live Demo:
See the Pen JavaScript - Find a pair of elements from an specified array whose sum equals a specific target number - array-ex- 26 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that finds a pair of indices in an array whose corresponding values sum to a target using a hash map.
- Write a JavaScript function that iterates over an array and returns the first pair of indices that meet the target sum condition.
- Write a JavaScript function that sorts the array and employs a two-pointer technique to find the target sum pair.
- Write a JavaScript function that handles duplicate elements and returns all unique index pairs whose sum equals the target.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to sort the following array of objects by title value.
Next: Write a JavaScript function to retrieve the value of a given property from all elements in an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.