JavaScript: Sort the specified array of objects by title value
JavaScript Array: Exercise-25 with Solution
Sort Objects by Title
Write a JavaScript function to sort the following array of objects by title value.
Sample object:
var library = [ { author: 'Bill Gates', title: 'The Road Ahead', libraryID: 1254}, { author: 'Steve Jobs', title: 'Walter Isaacson', libraryID: 4264}, { author: 'Suzanne Collins', title: 'Mockingjay: The Final Book of The Hunger Games', libraryID: 3245} ];
Visual Presentation:
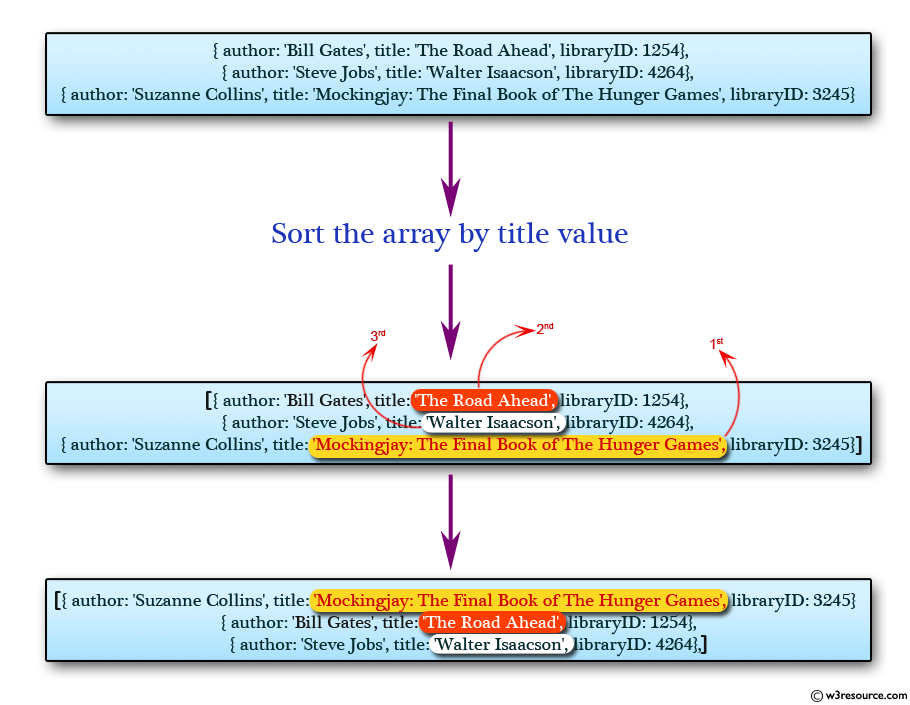
Sample Solution:
JavaScript Code:
// Array of book objects in the library
var library = [
{ author: 'Bill Gates', title: 'The Road Ahead', libraryID: 1254},
{ author: 'Steve Jobs', title: 'Walter Isaacson', libraryID: 4264},
{ author: 'Suzanne Collins', title: 'Mockingjay: The Final Book of The Hunger Games', libraryID: 3245}
];
// Function to compare two book objects based on their titles for sorting
function compare_to_sort(x, y) {
// Check if the title of book x is less than the title of book y
if (x.title < y.title)
return -1;
// Check if the title of book x is greater than the title of book y
if (x.title > y.title)
return 1;
// If titles are equal, return 0
return 0;
}
// Output the sorted library array based on the compare_to_sort function
console.log(library.sort(compare_to_sort));
Output:
[{"author":"Suzanne Collins","title":"Mockingjay: The Final Book of The Hunger Games","libraryID":3245},{"author":"Bill Gates","title":"The Road Ahead","libraryID":1254},{"author":"Steve Jobs","title":"Walter Isaacson","libraryID":4264}]
Flowchart:
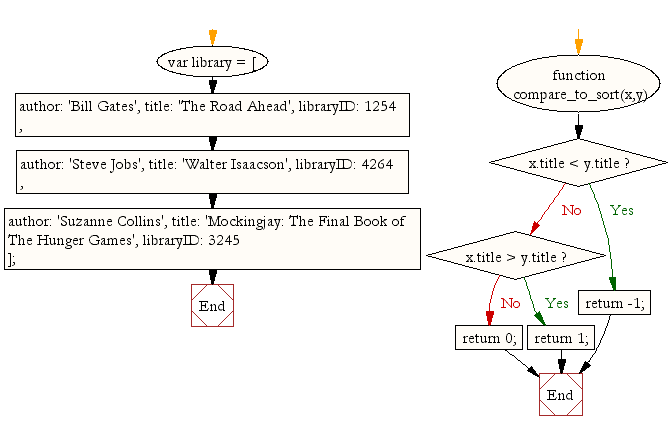
ES6 Version:
// Array of book objects in the library
const library = [
{ author: 'Bill Gates', title: 'The Road Ahead', libraryID: 1254},
{ author: 'Steve Jobs', title: 'Walter Isaacson', libraryID: 4264},
{ author: 'Suzanne Collins', title: 'Mockingjay: The Final Book of The Hunger Games', libraryID: 3245}
];
// Function to compare two book objects based on their titles for sorting
const compare_to_sort = (x, y) => {
// Check if the title of book x is less than the title of book y
if (x.title < y.title)
return -1;
// Check if the title of book x is greater than the title of book y
if (x.title > y.title)
return 1;
// If titles are equal, return 0
return 0;
};
// Output the sorted library array based on the compare_to_sort function
console.log(library.sort(compare_to_sort));
Live Demo:
See the Pen JavaScript - Sort the specified array of objects by title value - array-ex- 25 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that sorts an array of objects based on the title property alphabetically.
- Write a JavaScript function that uses a custom comparator to sort objects by title in a case-insensitive manner.
- Write a JavaScript function that sorts objects by title and, if equal, sorts by a secondary property like libraryID.
- Write a JavaScript function that handles objects missing the title property gracefully when sorting.
Go to:
PREV : Remove Falsy Values.
NEXT : Find Pair with Target Sum.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.