JavaScript: Find the difference of two arrays
JavaScript Array: Exercise-23 with Solution
Difference Between Arrays
Write a JavaScript function to find the difference between two arrays.
Test Data:
console.log(difference([1, 2, 3], [100, 2, 1, 10]));
["3", "10", "100"]
console.log(difference([1, 2, 3, 4, 5], [1, [2], [3, [[4]]],[5,6]]));
["6"]
console.log(difference([1, 2, 3], [100, 2, 1, 10]));
["3", "10", "100"]
Visual Presentation:
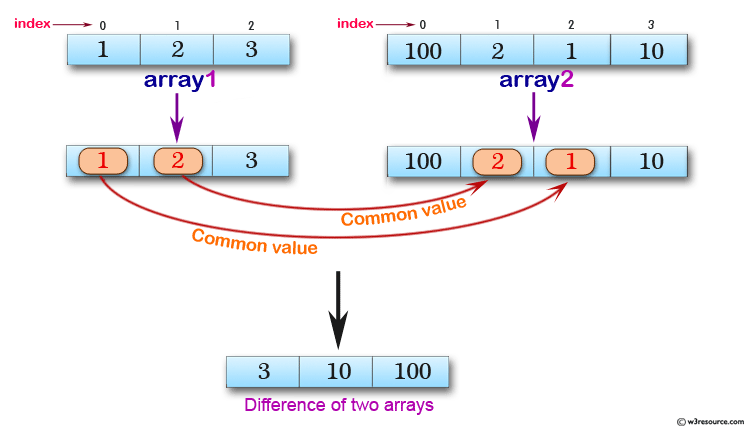
Sample Solution:
JavaScript Code:
// Function to find the difference of two arrays
function differenceOf2Arrays(array1, array2) {
// Temporary array to store the differences
var temp = [];
// Convert array1 to an array of numbers
array1 = array1.toString().split(',').map(Number);
// Convert array2 to an array of numbers
array2 = array2.toString().split(',').map(Number);
// Iterate through each element in array1
for (var i in array1) {
// If the element is not found in array2, add it to the temp array
if (array2.indexOf(array1[i]) === -1)
temp.push(array1[i]);
}
// Iterate through each element in array2
for (i in array2) {
// If the element is not found in array1, add it to the temp array
if (array1.indexOf(array2[i]) === -1)
temp.push(array2[i]);
}
// Return the temp array sorted in ascending order
return temp.sort((a, b) => a - b);
}
// Output the result of the differenceOf2Arrays function with sample arrays
console.log(differenceOf2Arrays([1, 2, 3], [100, 2, 1, 10]));
console.log(differenceOf2Arrays([1, 2, 3, 4, 5], [1, [2], [3, [[4]]], [5, 6]]));
Output:
[3,10,100] [6]
Flowchart:
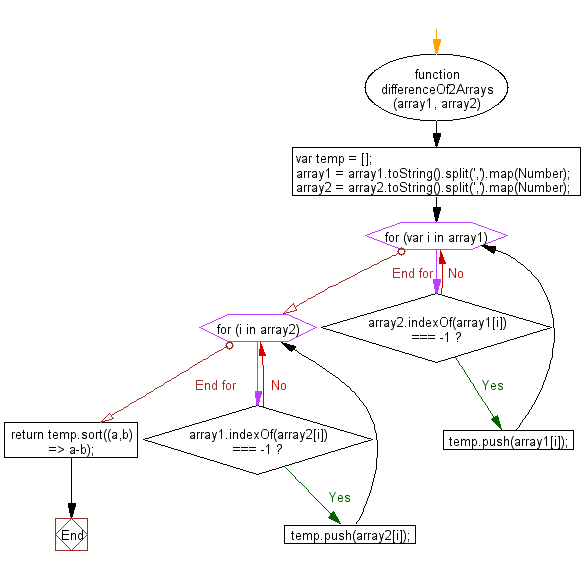
ES6 Version:
// Function to find the difference of two arrays
const differenceOf2Arrays = (array1, array2) => {
// Temporary array to store the differences
let temp = [];
// Convert array1 to an array of numbers
array1 = array1.toString().split(',').map(Number);
// Convert array2 to an array of numbers
array2 = array2.toString().split(',').map(Number);
// Iterate through each element in array1
for (let i of array1) {
// If the element is not found in array2, add it to the temp array
if (array2.indexOf(i) === -1)
temp.push(i);
}
// Iterate through each element in array2
for (let i of array2) {
// If the element is not found in array1, add it to the temp array
if (array1.indexOf(i) === -1)
temp.push(i);
}
// Return the temp array sorted in ascending order
return temp.sort((a, b) => a - b);
};
// Output the result of the differenceOf2Arrays function with sample arrays
console.log(differenceOf2Arrays([1, 2, 3], [100, 2, 1, 10]));
console.log(differenceOf2Arrays([1, 2, 3, 4, 5], [1, [2], [3, [[4]]], [5, 6]]));
Live Demo:
See the Pen JavaScript - Find the difference of two arrays - array-ex- 23 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program to compute the union of two arrays.
Next: Write a JavaScript function to remove. 'null', '0', '""', 'false', 'undefined' and 'NaN' values from an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics