JavaScript: Flatten a nested array
JavaScript Array: Exercise-21 with Solution
Flatten Nested Array
Write a JavaScript program to flatten a nested (any depth) array. If you pass shallow, the array will only be flattened to a single level.
Sample Data:
console.log(flatten([1, [2], [3, [[4]]],[5,6]]));
[1, 2, 3, 4, 5, 6]
console.log(flatten([1, [2], [3, [[4]]],[5,6]], true));
[1, 2, 3, [[4]], 5, 6]
Sample Solution:
JavaScript Code:
// Function to flatten a nested array
var flatten = function(a, shallow, r) {
// If the result array (r) is not provided, initialize it as an empty array
if (!r) {
r = [];
}
// If shallow is true, use concat.apply to flatten the array
if (shallow) {
return r.concat.apply(r, a);
}
// Iterate through each element in the array
for (var i = 0; i < a.length; i++) {
// Check if the current element is an array
if (a[i].constructor == Array) {
// Recursively flatten nested arrays
flatten(a[i], shallow, r);
} else {
// If the current element is not an array, push it to the result array
r.push(a[i]);
}
}
// Return the flattened array
return r;
}
// Output the result of the flatten function with a nested array
console.log(flatten([1, [2], [3, [[4]]], [5, 6]]));
// Output the result of the flatten function with a nested array using shallow flattening
console.log(flatten([1, [2], [3, [[4]]], [5, 6]], true));
Output:
[1,2,3,4,5,6] [1,2,3,[[4]],5,6]
Flowchart:
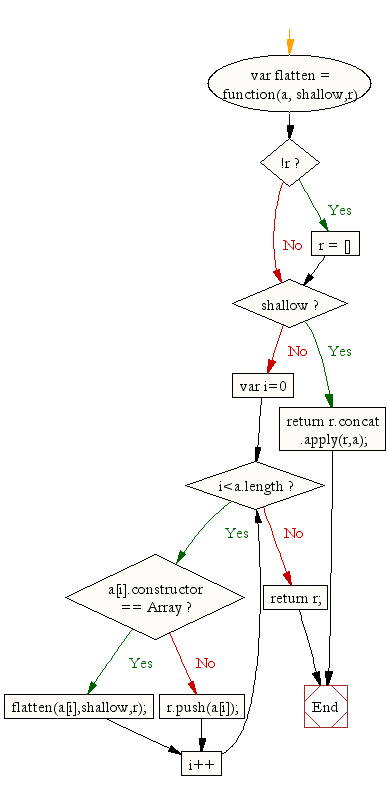
ES6 Version:
// Function to flatten a nested array
const flatten = (a, shallow, r = []) => {
// If shallow is true, use concat and spread syntax to flatten the array
if (shallow) {
return [...r, ...[].concat(...a)];
}
// Iterate through each element in the array
for (let i = 0; i < a.length; i++) {
// Check if the current element is an array
if (Array.isArray(a[i])) {
// Recursively flatten nested arrays
flatten(a[i], shallow, r);
} else {
// If the current element is not an array, push it to the result array
r.push(a[i]);
}
}
// Return the flattened array
return r;
};
// Output the result of the flatten function with a nested array
console.log(flatten([1, [2], [3, [[4]]], [5, 6]]));
// Output the result of the flatten function with a nested array using shallow flattening
console.log(flatten([1, [2], [3, [[4]]], [5, 6]], true));
Live Demo:
See the Pen JavaScript - Flatten a nested array - array-ex- 21 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that recursively flattens an array of arbitrary depth into a one-dimensional array.
- Write a JavaScript function that flattens an array one level deep when a shallow flag is provided.
- Write a JavaScript function that uses the reduce() method to flatten an array with both deep and shallow options.
- Write a JavaScript function that validates the input and gracefully handles non-array elements during flattening.
Go to:
PREV : Find Duplicate Values.
NEXT : Union of Two Arrays.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.