JavaScript: Find duplicate values in a array
JavaScript Array: Exercise-20 with Solution
Find Duplicate Values
Write a JavaScript program to find duplicate values in a JavaScript array.
Sample Solution:
JavaScript Code:
// Function to find duplicates in an array
function find_duplicate_in_array(arra1) {
// Object to store the count of each element in the array
var object = {};
// Array to store the elements with duplicates
var result = [];
// Iterate through each element in the array
arra1.forEach(function (item) {
// Check if the element is not in the object, initialize its count to 0
if (!object[item])
object[item] = 0;
// Increment the count of the current element
object[item] += 1;
})
// Iterate through the properties of the object
for (var prop in object) {
// Check if the count of the element is greater than or equal to 2
if (object[prop] >= 2) {
// Add the element to the result array
result.push(prop);
}
}
// Return the array containing duplicate elements
return result;
}
// Output the result of the function with a sample array
console.log(find_duplicate_in_array([1, 2, -2, 4, 5, 4, 7, 8, 7, 7, 71, 3, 6]));
Output:
["4","7"]
Flowchart:
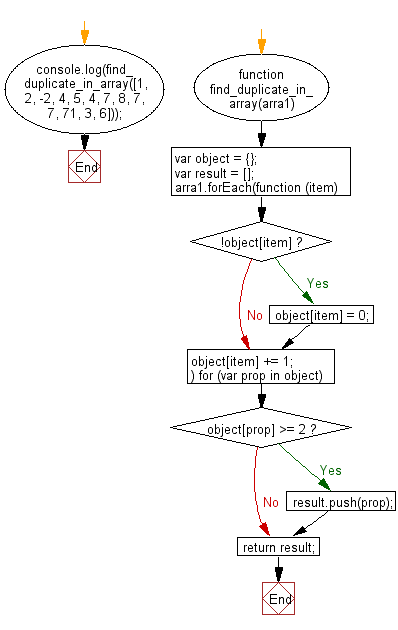
ES6 Version:
// Function to find duplicates in an array
const find_duplicate_in_array = (arra1) => {
// Object to store the count of each element in the array
const object = {};
// Array to store the elements with duplicates
const result = [];
// Iterate through each element in the array
arra1.forEach((item) => {
// Check if the element is not in the object, initialize its count to 0
if (!object[item]) {
object[item] = 0;
}
// Increment the count of the current element
object[item] += 1;
});
// Iterate through the properties of the object
for (const prop in object) {
// Check if the count of the element is greater than or equal to 2
if (object[prop] >= 2) {
// Add the element to the result array
result.push(prop);
}
}
// Return the array containing duplicate elements
return result;
};
// Output the result of the function with a sample array
console.log(find_duplicate_in_array([1, 2, -2, 4, 5, 4, 7, 8, 7, 7, 71, 3, 6]));
Live Demo:
See the Pen JavaScript - Find duplicate values in a array - array-ex- 20 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that identifies and returns duplicate values in an array using a frequency counter.
- Write a JavaScript function that filters an array to extract only elements that appear more than once.
- Write a JavaScript function that sorts and returns the duplicate elements found in an array.
- Write a JavaScript function that iterates through an array and collects duplicates without using built-in filtering methods.
Improve this sample solution and post your code through Disqus.
Previous: write a JavaScript program to compute the sum of each individual index value from the given arrays.
Next: Write a JavaScript program to flatten a nested (any depth) array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics