JavaScript: Compute the sum of each individual index value of two or more arrays
JavaScript Array: Exercise-19 with Solution
Sum of Arrays by Index
There are two arrays with individual values. Write a JavaScript program to compute the sum of each individual index value in the given array.
Sample array:
array1 = [1,0,2,3,4];
array2 = [3,5,6,7,8,13];
Expected Output:
[4, 5, 8, 10, 12, 13]
Sample Solution:
JavaScript Code:
// Function to calculate the sum of corresponding elements from two arrays
function Arrays_sum(array1, array2) {
// Initialize an empty array to store the sum of corresponding elements
var result = [];
// Initialize counters for iterating through the arrays
var ctr = 0;
var x = 0;
// Check if array1 is empty, return an error message if true
if (array1.length === 0)
return "array1 is empty";
// Check if array2 is empty, return an error message if true
if (array2.length === 0)
return "array2 is empty";
// Iterate through arrays until the end of either array is reached
while (ctr < array1.length && ctr < array2.length) {
// Calculate the sum of corresponding elements and push it to the result array
result.push(array1[ctr] + array2[ctr]);
// Increment the counter
ctr++;
}
// Check if array1 is exhausted
if (ctr === array1.length) {
// Append the remaining elements from array2 to the result array
for (x = ctr; x < array2.length; x++) {
result.push(array2[x]);
}
} else {
// Append the remaining elements from array1 to the result array
for (x = ctr; x < array1.length; x++) {
result.push(array1[x]);
}
}
// Return the resulting array
return result;
}
// Output the result of the function with sample arrays
console.log(Arrays_sum([1, 0, 2, 3, 4], [3, 5, 6, 7, 8, 13]));
Output:
[4,5,8,10,12,13]
Flowchart:
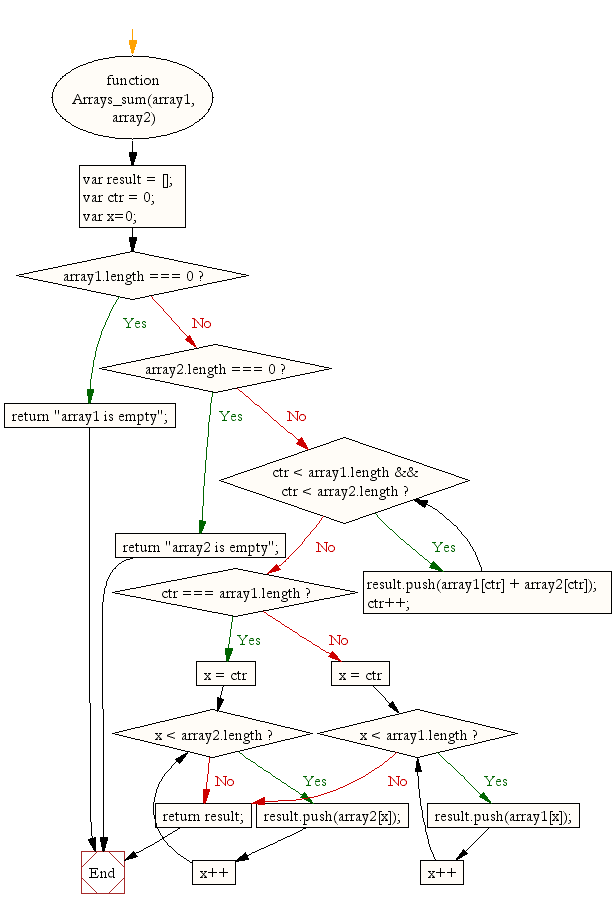
ES6 Version:
// Function to calculate the sum of corresponding elements from two arrays
const Arrays_sum = (array1, array2) => {
// Initialize an empty array to store the sum of corresponding elements
const result = [];
// Initialize counters for iterating through the arrays
let ctr = 0;
let x = 0;
// Check if array1 is empty, return an error message if true
if (array1.length === 0)
return "array1 is empty";
// Check if array2 is empty, return an error message if true
if (array2.length === 0)
return "array2 is empty";
// Iterate through arrays until the end of either array is reached
while (ctr < array1.length && ctr < array2.length) {
// Calculate the sum of corresponding elements and push it to the result array
result.push(array1[ctr] + array2[ctr]);
// Increment the counter
ctr++;
}
// Check if array1 is exhausted
if (ctr === array1.length) {
// Append the remaining elements from array2 to the result array
for (x = ctr; x < array2.length; x++) {
result.push(array2[x]);
}
} else {
// Append the remaining elements from array1 to the result array
for (x = ctr; x < array1.length; x++) {
result.push(array1[x]);
}
}
// Return the resulting array
return result;
};
// Output the result of the function with sample arrays
console.log(Arrays_sum([1, 0, 2, 3, 4], [3, 5, 6, 7, 8, 13]));
Live Demo:
See the Pen JavaScript - Compute the sum of each individual index value of two or more arrays - array-ex- 19 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that computes the element-wise sum of two arrays and returns the result as a new array.
- Write a JavaScript function that handles arrays of different lengths by treating missing elements as zero.
- Write a JavaScript function that uses the map() method to add corresponding elements of two arrays.
- Write a JavaScript function that iterates concurrently over two arrays with a for loop to compute the index-wise sum.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program to perform a binary search.
Next: Write a JavaScript program to find duplicate values in a JavaScript array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.