JavaScript: Find the leap years from a given range of years
JavaScript Array: Exercise-16 with Solution
Find the leap years in a given range of years.
Visual Presentation:
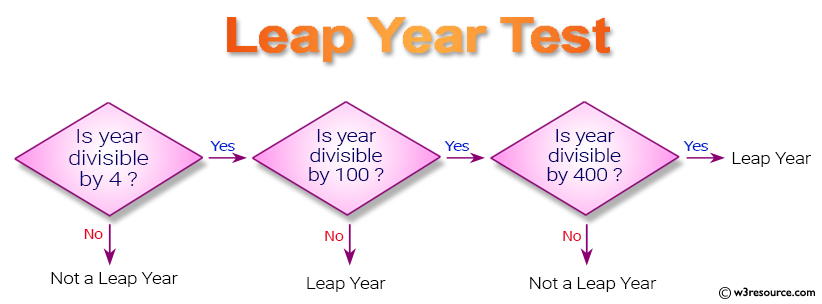
Sample Solution:
JavaScript Code:
// Function to generate an array of leap years within a specified range
function leap_year_range(st_year, end_year) {
// Initialize an array 'year_range' to store all years within the range
var year_range = [];
// Loop through the years in the specified range and add them to the array
for (var i = st_year; i <= end_year; i++) {
year_range.push(i);
}
// Initialize a new array 'new_array' to store leap years
var new_array = [];
// Use forEach to iterate through each year in 'year_range'
year_range.forEach(
function(year) {
// Call the 'test_LeapYear' function to check if the current year is a leap year
if (test_LeapYear(year)) {
// If it is a leap year, add it to 'new_array'
new_array.push(year);
}
}
);
// Return the array of leap years
return new_array;
}
// Function to test if a given year is a leap year
function test_LeapYear(year) {
// Check if the year is divisible by 4 but not divisible by 100, or if it is divisible by 100 and 400
if ((year % 4 === 0 && year % 100 !== 0) || (year % 100 === 0 && year % 400 === 0)) {
// Return the year if it's a leap year
return year;
} else {
// Return false if it's not a leap year
return false;
}
}
// Output the array of leap years in the range 2000 to 2012
console.log(leap_year_range(2000, 2012));
Output:
[2000,2004,2008,2012]
Flowchart:
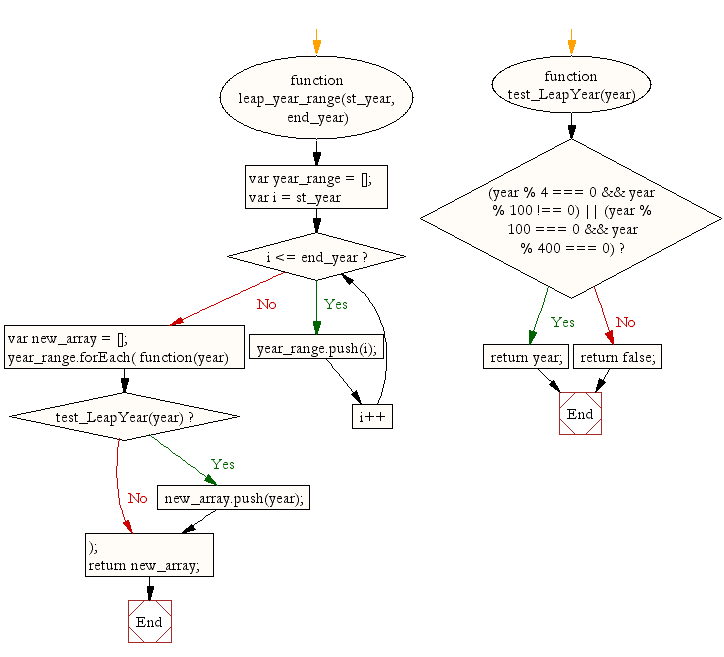
ES6 Version:
// Function to generate an array of leap years within a specified range
const leap_year_range = (st_year, end_year) => {
// Initialize an array 'year_range' to store all years within the range
const year_range = [];
// Loop through the years in the specified range and add them to the array
for (let i = st_year; i <= end_year; i++) {
year_range.push(i);
}
// Initialize a new array 'new_array' to store leap years
const new_array = [];
// Use forEach to iterate through each year in 'year_range'
year_range.forEach((year) => {
// Call the 'test_LeapYear' function to check if the current year is a leap year
if (test_LeapYear(year)) {
// If it is a leap year, add it to 'new_array'
new_array.push(year);
}
});
// Return the array of leap years
return new_array;
};
// Function to test if a given year is a leap year
const test_LeapYear = (year) => {
// Check if the year is divisible by 4 but not divisible by 100, or if it is divisible by 100 and 400
if ((year % 4 === 0 && year % 100 !== 0) || (year % 100 === 0 && year % 400 === 0)) {
// Return the year if it's a leap year
return year;
} else {
// Return false if it's not a leap year
return false;
}
};
// Output the array of leap years in the range 2000 to 2012
console.log(leap_year_range(2000, 2012));
Live Demo:
See the Pen JavaScript - Find the leap years from a given range of years - array-ex- 16 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program to the colors entered in an array by a specific format.
Next: Write a JavaScript program to shuffle an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-array-exercise-16.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics