JavaScript: Display the colors entered in an array by a specific format
JavaScript Array: Exercise-15 with Solution
Display Colors with Ordinals
Write a JavaScript program to display the colors in the following way.
Here is the sample array:
color = ["Blue ", "Green", "Red", "Orange", "Violet", "Indigo", "Yellow "];
o = ["th","st","nd","rd"]
Output
"1st choice is Blue ."
"2nd choice is Green."
"3rd choice is Red."
- - - - - - - - - - - - -
Sample Solution:
JavaScript Code:
// Declare an array 'color' containing color names
var color = ["Blue ", "Green", "Red", "Orange", "Violet", "Indigo", "Yellow "];
// Function to generate ordinal numbers (1st, 2nd, 3rd, etc.)
function Ordinal(n) {
// Define an array 'o' for suffixes of ordinal numbers
var o = ["th", "st", "nd", "rd"],
x = n % 100;
// Return the ordinal number with the appropriate suffix
return x + (o[(x - 20) % 10] || o[x] || o[0]);
}
// Loop through each element in the 'color' array
for (n = 0; n < color.length; n++) {
// Calculate the ordinal number for the current position
var ordinal = n + 1;
// Create a string with the ordinal number, color choice, and a period
var output = (Ordinal(ordinal) + " choice is " + color[n] + ".");
// Output the string to the console
console.log(output);
}
Output:
1st choice is Blue . 2nd choice is Green. 3rd choice is Red. 4th choice is Orange. 5th choice is Violet. 6th choice is Indigo. 7th choice is Yellow .
Flowchart:
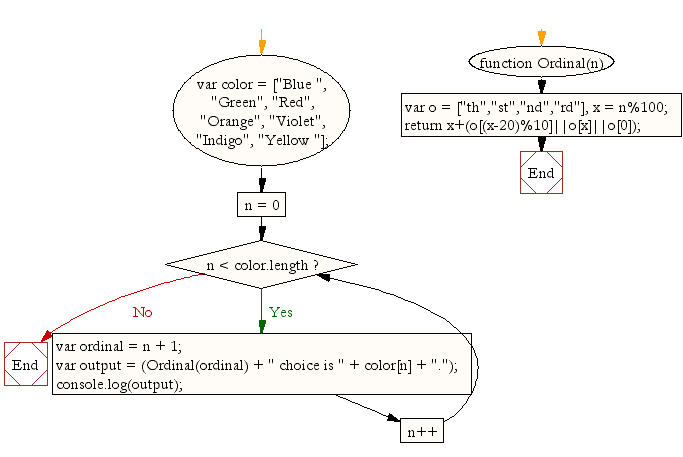
ES6 Version:
// Declare an array 'color' containing color names
const color = ["Blue ", "Green", "Red", "Orange", "Violet", "Indigo", "Yellow "];
// Function to generate ordinal numbers (1st, 2nd, 3rd, etc.)
const Ordinal = (n) => {
// Define an array 'o' for suffixes of ordinal numbers
const o = ["th", "st", "nd", "rd"];
const x = n % 100;
// Return the ordinal number with the appropriate suffix
return x + (o[(x - 20) % 10] || o[x] || o[0]);
};
// Loop through each element in the 'color' array using forEach
color.forEach((_, index) => {
// Calculate the ordinal number for the current position
const ordinal = index + 1;
// Create a string with the ordinal number, color choice, and a period
const output = `${Ordinal(ordinal)} choice is ${color[index]}.`;
// Output the string to the console
console.log(output);
});
Live Demo:
See the Pen JavaScript - Display the colors entered in an array by a specific format - array-ex- 15 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program to remove duplicate items from an array (ignore case sensitivity).
Next: Write a JavaScript program to find the leap years from a given range of years.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics