JavaScript: Remove duplicate items from an array, ignore case sensitivity
JavaScript Array: Exercise-14 with Solution
Remove Duplicates
Write a JavaScript program to remove duplicate items from an array (ignore case sensitivity).
Visual Presentation:
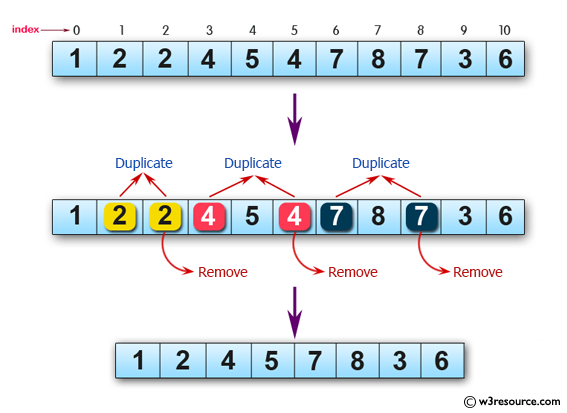
Sample Solution-1:
JavaScript Code:
// Function to remove duplicate elements from an array
function removeDuplicates(num) {
// Initialize variables: x for iteration, len for array length, out for the result array, obj for object to track unique elements
var x,
len = num.length,
out = [],
obj = {};
// Iterate through the input array and add each element to the object with a value of 0
for (x = 0; x < len; x++) {
obj[num[x]] = 0;
}
// Iterate through the object and push each unique element to the result array
for (x in obj) {
out.push(x);
}
// Return the array containing unique elements
return out;
}
// Initialize an array with duplicate elements
var Mynum = [1, 2, 2, 4, 5, 4, 7, 8, 7, 3, 6];
// Call the removeDuplicates function with the array and store the result
var result = removeDuplicates(Mynum);
// Output the original array and the result array without duplicates
console.log(Mynum);
console.log(result);
Sample Output:
[1,2,2,4,5,4,7,8,7,3,6] ["1","2","3","4","5","6","7","8"]
Flowchart:
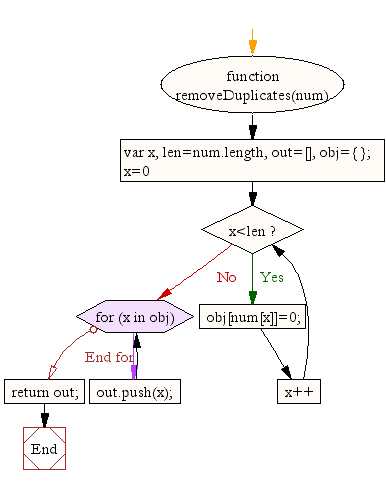
Sample Solution-2:
Removing duplicates from an array in JavaScript can be done in a variety of ways, such as using Array.prototype.reduce(), Array.prototype.filter() or even a simple for loop. But there's an easier alternative. JavaScript's built-in Set object is described as a collection of values, where each value may occur only once. A Set object is also iterable, making it easily convertible to an array using the spread (...) operator.
JavaScript Code:
// Source: https://bit.ly/3hEZdCl
// Remove duplicates from a JavaScript array using the Set data structure
// Define an array 'nums' with duplicate elements
const nums = [1, 2, 2, 3, 1, 2, 4, 5, 4, 2, 6];
// Create a new Set from the array to automatically remove duplicates
const uniqueNumsSet = new Set(nums);
// Convert the Set back to an array using the spread operator
const uniqueNumsArray = [...uniqueNumsSet];
// Output the array with duplicates removed
console.log(uniqueNumsArray);
Output:
[1,2,3,4,5,6]
Live Demo:
See the Pen JavaScript - Remove duplicate items from an array, ignore case sensitivity - array-ex- 14 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that removes duplicate items from an array using a Set and returns the unique elements.
- Write a JavaScript function that iterates through an array and constructs a new array containing only unique values.
- Write a JavaScript function that filters duplicates from an array with mixed data types based on strict equality.
- Write a JavaScript function that uses a hash map to record frequencies and then removes duplicates accordingly.
Contribute your code and comments through Disqus.
Previous: Write a JavaScript program to add items in an blank array and display the items.
Next: Write a JavaScript program to display the colors entered in an array by a specific format.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.