JavaScript: Compute the sum and product of an array of integers
JavaScript Array: Exercise-12 with Solution
Sum and Product of Array
Write a JavaScript program to compute the sum and product of an array of integers.
Visual Presentation:
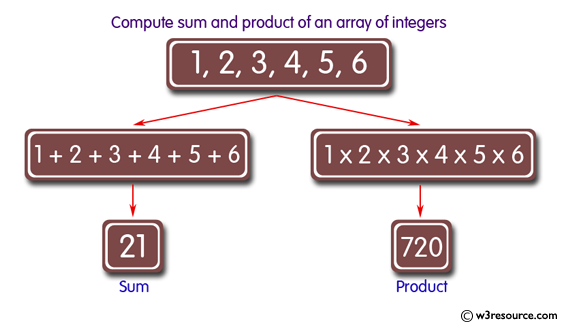
Sample Solution:
JavaScript Code:
// Declare and initialize an array of numbers
var array = [1, 2, 3, 4, 5, 6];
// Initialize variables for sum (s) and product (p)
var s = 0;
var p = 1;
// Iterate through the array using a for loop
for (var i = 0; i < array.length; i += 1) {
// Add the current element to the sum
s += array[i];
// Multiply the current element to the product
p *= array[i];
}
// Output the calculated sum and product
console.log('Sum : ' + s + ' Product : ' + p);
Output:
Sum : 21 Product : 720
Flowchart:
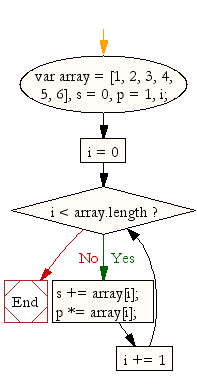
ES6 Version:
// Declare and initialize an array of numbers
const array = [1, 2, 3, 4, 5, 6];
// Initialize variables for sum (s) and product (p)
let s = 0;
let p = 1;
// Iterate through the array using a for...of loop
for (const element of array) {
// Add the current element to the sum
s += element;
// Multiply the current element to the product
p *= element;
}
// Output the calculated sum and product
console.log(`Sum : ${s} Product : ${p}`);
Live Demo:
See the Pen avaScript - sum and product of an array of integers- array-ex- 12 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that computes both the sum and product of all numbers in an array and returns them as an object.
- Write a JavaScript function that uses a for loop to calculate the sum and product simultaneously.
- Write a JavaScript function that employs the reduce() method twice to obtain the sum and product separately.
- Write a JavaScript function that validates that all elements are numbers before computing their sum and product.
Go to:
PREV : Sum of Squares in Array.
NEXT : Add Items to Array.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.