JavaScript: Get the first element of an array
JavaScript Array: Exercise-1 with Solution
Check Array Input
Write a JavaScript function to check whether an 'input' is an array or not.
Test Data:
console.log(is_array('w3resource'));
console.log(is_array([1, 2, 4, 0]));
false
true
Sample Solution:
JavaScript Code:
// Function to check if the input is an array
var is_array = function(input) {
// Using toString method to get the class of the input and checking if it is "[object Array]"
if (toString.call(input) === "[object Array]")
// Return true if the input is an array
return true;
// Return false if the input is not an array
return false;
};
// Testing the function with a string
console.log(is_array('w3resource'));
// Testing the function with an array
console.log(is_array([1, 2, 4, 0]));
Output:
false true
Flowchart:
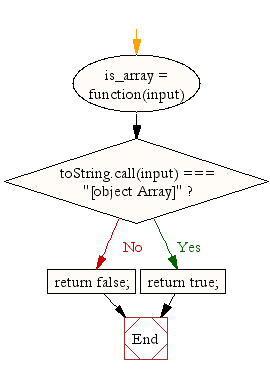
ES6 Version:
// Function to check if the input is an array
const is_array = input => {
// Using toString method to get the class of the input and checking if it is "[object Array]"
if (toString.call(input) === "[object Array]") {
// Return true if the input is an array
return true;
}
// Return false if the input is not an array
return false;
};
// Testing the function with a string
console.log(is_array('w3resource'));
// Testing the function with an array
console.log(is_array([1, 2, 4, 0]));
Live Demo:
See the Pen JavaScript - Get the first element of an array- array-ex-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Javascript Array Exercises
Next: Write a JavaScript function to clone an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics