JavaScript : Filter an array of objects based on a condition while also filtering out unspecified keys
JavaScript fundamental (ES6 Syntax): Exercise-98 with Solution
Write a JavaScript program to filter an array of objects based on a condition while also filtering out unspecified keys.
- Use Array.prototype.filter() to filter the array based on the predicate fn so that it returns the objects for which the condition returned a truthy value.
- On the filtered array, use Array.prototype.map() to return the new object.
- Use Array.prototype.reduce() to filter out the keys which were not supplied as the keys argument.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'reducedFilter' function to filter an array of objects and return a new array of objects containing only selected keys based on a filtering function.
const reducedFilter = (data, keys, fn) =>
// Filter the data array based on the filtering function 'fn', then map the filtered objects to return a new array of objects containing only selected keys.
data.filter(fn).map(el =>
// Reduce the selected keys for each object to build a new object containing only those selected keys.
keys.reduce((acc, key) => {
acc[key] = el[key];
return acc;
}, {})
);
// Test the 'reducedFilter' function with sample data.
const data = [
{
id: 1,
name: 'john',
age: 24
},
{
id: 2,
name: 'mike',
age: 50
}
];
console.log(reducedFilter(data, ['id', 'name'], item => item.age > 24)); // Output: [{ id: 2, name: 'mike' }]
Output:
[{"id":2,"name":"mike"}]
Flowchart:
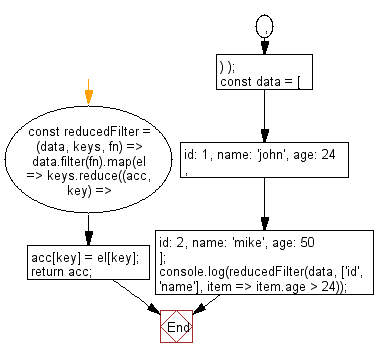
Live Demo:
See the Pen javascript-basic-exercise-98-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to create an object composed of the properties the given function returns truthy for. The function is invoked with two arguments: (value, key).
Next: Write a JavaScript program to hash an given input string into a whole number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-98.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics