JavaScript: Pick the key-value pairs corresponding to the given keys from an object
JavaScript fundamental (ES6 Syntax): Exercise-96 with Solution
Pick Keys from Object
Write a JavaScript program to pick the key-value pairs corresponding to the given keys from an object.
- Use Array.prototype.reduce() to convert the filtered/picked keys back to an object with the corresponding key-value pairs if the key exists in the object.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'pick' function to pick specific key-value pairs from an object.
const pick = (obj, arr) =>
// Reduce the array of keys 'arr' to build a new object containing only the specified keys.
arr.reduce((acc, curr) => (curr in obj && (acc[curr] = obj[curr]), acc), {});
// Test the 'pick' function with an object and an array of keys to pick.
console.log(pick({ a: 1, b: '2', c: 3 }, ['a', 'c'])); // Output: { a: 1, c: 3 }
Output:
{"a":1,"c":3}
Visual Presentation:
Flowchart:
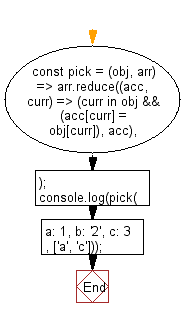
Live Demo:
See the Pen javascript-basic-exercise-96-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that creates a new object containing only the specified keys from an input object.
- Write a JavaScript function that accepts an object and an array of keys, then returns a subset object with those key-value pairs.
- Write a JavaScript program that uses destructuring to extract selected properties from an object and recombines them.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to add an event listener to an element with the ability to use event delegation.
Next: Write a JavaScript program to create an object composed of the properties the given function returns truthy for. The function is invoked with two arguments: (value, key).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.