JavaScript: Remove an event listener from an element
JavaScript fundamental (ES6 Syntax): Exercise-93 with Solution
Remove Event Listener from Element
Write a JavaScript program to remove an event listener from an element.
- Use EventTarget.removeEventListener() to remove an event listener from an element.
- Omit the fourth argument opts to use false or specify it based on the options used when the event listener was added.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'off' function to remove an event listener from an element.
const off = (el, evt, fn, opts = false) => el.removeEventListener(evt, fn, opts);
// Define a function 'fn' to log an exclamation mark to the console.
const fn = () => console.log('!');
// Add an event listener for 'click' events on the document body, calling the 'fn' function.
document.body.addEventListener('click', fn);
// Remove the event listener for 'click' events on the document body that calls the 'fn' function.
console.log(off(document.body, 'click', fn));
Output:
undefined
Flowchart:
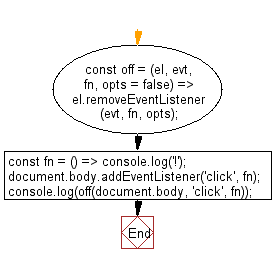
Live Demo:
See the Pen javascript-basic-exercise-93-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to create a function that gets the argument at index n. If n is negative, the nth argument from the end is returned.
Next: Write a JavaScript program to move the specified amount of elements to the end of the array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics