JavaScript: Create a function that gets the argument at index n. If n is negative, the nth argument from the end is returned
JavaScript fundamental (ES6 Syntax): Exercise-92 with Solution
Get nth Argument by Index or Reverse Index
Write a JavaScript program to create a function that gets the argument at index n. If n is negative, the nth argument from the end is returned.
- Use Array.prototype.slice() to get the desired argument at index n.
- If n is negative, the nth argument from the end is returned.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'nthArg' function to return a function that retrieves the nth argument passed to it.
const nthArg = n => (...args) => args.slice(n)[0];
// Create a function 'third' that retrieves the third argument passed to it.
const third = nthArg(2);
third(1, 2, 3); // Output: 3 (third argument)
third(1, 2); // Output: undefined (no third argument)
// Create a function 'last' that retrieves the last argument passed to it.
const last = nthArg(-1);
console.log(last(1, 2, 3, 4, 5)); // Output: 5 (last argument)
Output:
5
Flowchart:
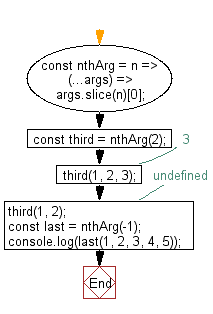
Live Demo:
See the Pen javascript-basic-exercise-92-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that returns the nth argument from a function call, with support for negative indices indicating positions from the end.
- Write a JavaScript function that extracts an argument at a specified index from the arguments object, handling negative indexes.
- Write a JavaScript program that uses rest parameters to access the nth parameter, even when n is negative, to return the desired value.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program that will return true if the provided predicate function returns false for all elements in a collection, false otherwise.
Next: Write a JavaScript program to remove an event listener from an element.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.