JavaScript: Return true if the provided predicate function returns false for all elements in a collection, false otherwise
JavaScript fundamental (ES6 Syntax): Exercise-91 with Solution
All Elements Fail Predicate Check
Write a JavaScript program that returns true if the provided predicate function returns false for all elements in a collection, false otherwise.
- Use Array.prototype.some() to test if any elements in the collection return true based on fn.
- Omit the second argument, fn, to use Boolean as a default.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'none' function to check if none of the elements in an array satisfy a given condition.
const none = (arr, fn = Boolean) => !arr.some(fn);
// Example usage:
console.log(none([0, 1, 3, 0], x => x == 2));
console.log(none([0, 0, 0])); //
Output:
true true
Flowchart:
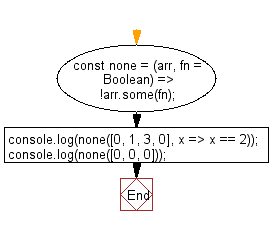
Live Demo:
See the Pen javascript-basic-exercise-91-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that returns true if a provided predicate function returns false for every element in a collection.
- Write a JavaScript function that iterates over an array and confirms that no element satisfies a given condition.
- Write a JavaScript program that uses Array.every() with the negation of a predicate to determine if all elements fail the test.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to nest a given flat array of objects linked to one another recursively.
Next: Write a JavaScript program to create a function that gets the argument at index n. If n is negative, the nth argument from the end is returned.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.