JavaScript: Combine the numbers of a given array into an array containing all combinations
JavaScript fundamental (ES6 Syntax): Exercise-9 with Solution
Write a JavaScript program to combine the numbers of a given array into an array containing all combinations.
- Use Array.prototype.reduce() combined with Array.prototype.map() to iterate over elements and combine into an array containing all combinations.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function called `powerset` that takes an array `arr`.
const powerset = arr =>
// Reduce the array to generate the powerset.
arr.reduce(
// For each element in the array, concatenate it with each element of the accumulated result.
(a, v) => a.concat(a.map(r => [v].concat(r))),
[[]]
);
// Test cases
console.log(powerset([1, 2]));
console.log(powerset([1, 2, 3]));
console.log(powerset([1, 2, 3, 4]));
Output:
[[],[1],[2],[2,1]] [[],[1],[2],[2,1],[3],[3,1],[3,2],[3,2,1]] [[],[1],[2],[2,1],[3],[3,1],[3,2],[3,2,1],[4],[4,1],[4,2],[4,2,1],[4,3],[4,3,1],[4,3,2],[4,3,2,1]]
Visual Presentation:
Flowchart:
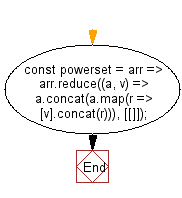
Live Demo:
See the Pen javascript-basic-exercise-1-9 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to filter out the specified values from an specified array. Return the original array without the filtered values.
Next: Write a JavaScript program to extract out the values at the specified indexes from an specified array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-9.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics