JavaScript: Map the values of an array to an object using a function
JavaScript: Exercise-82 with Solution
Write a JavaScript program to map array values to an object using a function. The key-value pairs consist of the original value as the key and the mapped value.
Note: Use an anonymous inner function scope to declare an undefined memory space, using closures to store a return value. Use a new Array to store the array with a map of the function over its data set and a comma operator to return a second step, without needing to move from one context to another (due to closures and order of operations).
- Use Array.prototype.reduce() to apply fn to each element in arr and combine the results into an object.
- Use el as the key for each property and the result of fn as the value.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the function 'mapObject' to map an array to an object using a given function.
const mapObject = (arr, fn) =>
// Immediately invoked function expression (IIFE) to avoid creating unnecessary variable assignments.
(a => (
// Create an array where the first element is the original array and the second element is the result of applying the function to each element.
(a = [arr, arr.map(fn)]),
// Reduce the original array, mapping each element to its corresponding transformed value and constructing a new object.
a[0].reduce((acc, val, ind) => ((acc[val] = a[1][ind]), acc), {})
))();
// Define a function 'squareIt' that squares each element of an array and returns the result as an object.
const squareIt = arr => mapObject(arr, a => a * a);
// Example usage:
console.log(squareIt([1, 2, 3])); // Outputs: { '1': 1, '2': 4, '3': 9 }
Output:
{"1":1,"2":4,"3":9}
Visual Presentation:
Flowchart:
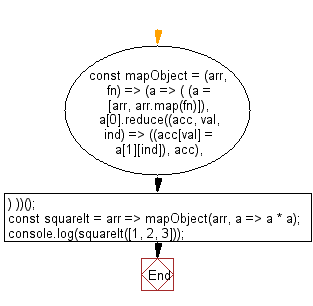
Live Demo:
See the Pen javascript-basic-exercise-82-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to create an object with keys generated by running the provided function for each key and the same values as the provided object.
Next: Write a JavaScript program to create a new string with the results of calling a provided function on every character in the calling string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-82.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics