JavaScript: Create an object with keys generated by running the provided function for each key and the same values as the provided object
JavaScript fundamental (ES6 Syntax): Exercise-81 with Solution
Write a JavaScript program to create an object with keys generated by running the provided function for each key. The object will have the same values as the provided object.
- Use Object.keys() to iterate over the object's keys.
- Use Array.prototype.reduce() to create a new object with the same values and mapped keys using fn.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the function 'mapKeys' to map the keys of an object using a given function.
const mapKeys = (obj, fn) =>
// Reduce the keys of the object to a new object, applying the function to each key and assigning the original value.
Object.keys(obj).reduce((acc, k) => {
// Assign the value of the original key to the new key generated by applying the function.
acc[fn(obj[k], k, obj)] = obj[k];
return acc;
}, {});
// Example usage:
console.log(mapKeys({ a: 1, b: 2 }, (val, key) => key + val)); // Outputs: { 'a1': 1, 'b2': 2 }
Output:
{"a1":1,"b2":2}
Flowchart:
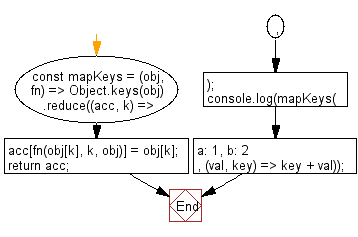
Live Demo:
See the Pen javascript-basic-exercise-81-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to implement the Luhn Algorithm used to validate a variety of identification numbers, such as credit card numbers, IMEI numbers, National Provider Identifier numbers etc.
Next: Write a JavaScript program to map the values of an array to an object using a function, where the key-value pairs consist of the original value as the key and the mapped value.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics