JavaScript: Implement the Luhn Algorithm used to validate a variety of identification numbers
JavaScript fundamental (ES6 Syntax): Exercise-80 with Solution
Validate with Luhn Algorithm
Write a JavaScript program to implement the Luhn Algorithm used to validate identification numbers. For example, credit card numbers, IMEI numbers, National Provider Identifier numbers etc.
- Use String.prototype.split(''), Array.prototype.reverse() and Array.prototype.map() in combination with parseInt() to obtain an array of digits.
- Use Array.prototype.splice(0, 1) to obtain the last digit.
- Use Array.prototype.reduce() to implement the Luhn Algorithm.
- Return true if sum is divisible by 10, false otherwise.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the function 'luhnCheck' to perform the Luhn algorithm check on a given number.
const luhnCheck = num => {
// Convert the number to a string, split it into an array of digits, reverse the array, and convert each digit to an integer.
let arr = (num + '').split('').reverse().map(x => parseInt(x));
// Extract the last digit from the array.
let lastDigit = arr.splice(0, 1)[0];
// Calculate the Luhn sum using the Luhn algorithm.
let sum = arr.reduce((acc, val, i) => (i % 2 !== 0 ? acc + val : acc + ((val * 2) % 9) || 9), 0);
// Add the last digit to the sum.
sum += lastDigit;
// Check if the sum is divisible by 10.
return sum % 10 === 0;
};
// Example usages:
console.log(luhnCheck('4485275742308327'));
console.log(luhnCheck(6011329933655299));
console.log(luhnCheck(123456789));
Output:
true false false
Flowchart:
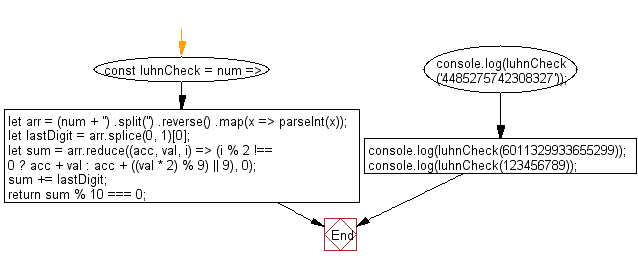
Live Demo:
See the Pen javascript-basic-exercise-80-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that implements the Luhn Algorithm to validate a credit card number.
- Write a JavaScript function that checks if an identification number passes the Luhn checksum.
- Write a JavaScript program that processes a string of digits and returns true if it satisfies the Luhn formula.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to take any number of iterable objects or objects with a length property and returns the longest one.
Next: Write a JavaScript program to create an object with keys generated by running the provided function for each key and the same values as the provided object.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics