JavaScript: Take any number of iterable objects or objects with a length property and returns the longest one
JavaScript fundamental (ES6 Syntax): Exercise-79 with Solution
Find Longest Iterable Object
Write a JavaScript program to take any number of iterable objects or objects with a length property and return the longest one.
- Use Array.prototype.reduce(), comparing the length of objects to find the longest one.
- If multiple objects have the same length, the first one will be returned.
- Returns undefined if no arguments are provided.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the function 'longestItem' to find the longest item among the provided values.
const longestItem = (...vals) =>
// Spread the provided values into an array, sort the array by the length of each item in descending order,
// and return the first (longest) item.
[...vals].sort((a, b) => b.length - a.length)[0];
// Example usages:
console.log(longestItem('this', 'is', 'a', 'testcase')); // Outputs: 'testcase'
console.log(longestItem(...['a', 'ab', 'abc'])); // Outputs: 'abc'
console.log(longestItem(...['a', 'ab', 'abc'], 'abcd')); // Outputs: 'abcd'
console.log(longestItem([1, 2, 3], [1, 2], [1, 2, 3, 4, 5])); // Outputs: [1, 2, 3, 4, 5]
console.log(longestItem([1, 2, 3], 'foobar')); // Outputs: 'foobar'
Output:
testcase abc abcd [1,2,3,4,5] foobar
Visual Presentation:
Flowchart:
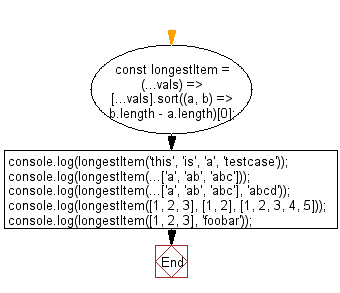
Live Demo:
See the Pen javascript-basic-exercise-79-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that takes multiple iterable objects and returns the one with the greatest length.
- Write a JavaScript function that compares objects with a length property and returns the longest among them.
- Write a JavaScript program that iterates over various arrays or strings and identifies the longest one based on length.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to invert the key-value pairs of an object, without mutating it. The corresponding inverted value of each inverted key is an array of keys responsible for generating the inverted value. If a function is supplied, it is applied to each inverted key.
Next: Write a JavaScript program to implement the Luhn Algorithm used to validate a variety of identification numbers, such as credit card numbers, IMEI numbers, National Provider Identifier numbers etc.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.