JavaScript: Iterate over all own properties of an object, running a callback for each one
JavaScript fundamental (ES6 Syntax): Exercise-77 with Solution
Iterate Over Object Properties
Write a JavaScript program to iterate over all the properties of an object, running a callback for each one.
- Use Object.keys(obj) to get all the properties of the object.
- Use Array.prototype.forEach() to run the provided function for each key-value pair.
- The callback receives three arguments - the value, the key and the object.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the function 'forOwn' to iterate over an object's own enumerable properties and apply a callback function to each property.
const forOwn = (obj, fn) =>
// Iterate over the keys of the object using Object.keys() and apply the callback function to each property.
Object.keys(obj).forEach(key => fn(obj[key], key, obj));
// Example usage:
forOwn({ foo: 'bar', a: 1 }, v => console.log(v)); // Outputs: 'bar', 1
Output:
bar 1
Flowchart:
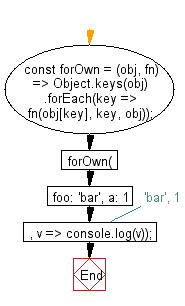
Live Demo:
See the Pen javascript-basic-exercise-76-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that iterates over all enumerable properties of an object and executes a callback for each.
- Write a JavaScript function that uses for...in to traverse an object and logs each key-value pair.
- Write a JavaScript program that collects an array of property names from an object while iterating over its properties.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to execute a provided function once for each array element, starting from the array's last element.
Next: Write a JavaScript program to invert the key-value pairs of an object, without mutating it. The corresponding inverted value of each inverted key is an array of keys responsible for generating the inverted value. If a function is supplied, it is applied to each inverted key.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.