JavaScript: Count the occurrences of a value in an array
JavaScript fundamental (ES6 Syntax): Exercise-70 with Solution
Count Value in Array
Write a JavaScript program to count a value in an array.
- Use Array.prototype.reduce() to increment a counter each time the specific value is encountered inside the array.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'countOccurrences' that counts the number of occurrences of a value in an array.
const countOccurrences = (arr, val) =>
arr.reduce((a, v) => (v === val ? a + 1 : a), 0);
// Example usages:
console.log(countOccurrences([1, 1, 2, 1, 2, 3], 1)); // Output: 3
console.log(countOccurrences([1, 1, 2, 1, 2, 3], 2)); // Output: 2
console.log(countOccurrences([1, 1, 2, 1, 2, 3], 3)); // Output: 1
Output:
3 2 1
Visual Presentation:
Flowchart:
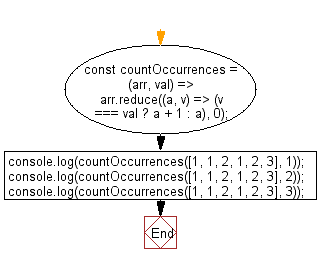
Live Demo:
See the Pen javascript-basic-exercise-70-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that counts the number of times a specific value appears in an array.
- Write a JavaScript function that uses reduce() to tally occurrences of a given element in an array.
- Write a JavaScript program that searches an array for a target value and returns its frequency count.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to group the elements of an array based on the given function and returns the count of elements in each group.
Next: Write a JavaScript program to create a deep clone of an object.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.