JavaScript: Group the elements of an array based on the given function and returns the count of elements in each group
JavaScript fundamental (ES6 Syntax): Exercise-69 with Solution
Group Array and Count Elements
Write a JavaScript program to group array elements based on the given function. It return the count of elements in each group.
- Use Array.prototype.map() to map the values of an array to a function or property name.
- Use Array.prototype.reduce() to create an object, where the keys are produced from the mapped results.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'countBy' that takes an array and a function (or property name) as arguments and returns an object.
const countBy = (arr, fn) =>
arr.map(typeof fn === 'function' ? fn : val => val[fn]) // Convert each element of the array to a key based on the function or property name.
.reduce((acc, val, i) => { // Reduce the mapped array to an object counting occurrences of each key.
acc[val] = (acc[val] || 0) + 1; // Increment the count for each key.
return acc;
}, {});
// Example usages:
console.log(countBy([6, 10, 100, 10], Math.sqrt));
console.log(countBy([6.1, 4.2, 6.3], Math.floor));
console.log(countBy(['one', 'two', 'three'], 'length'));
Output:
{"10":1,"2.449489742783178":1,"3.1622776601683795":2} {"4":1,"6":2} {"3":2,"5":1}
Flowchart:
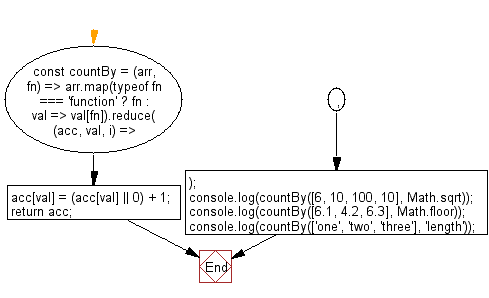
Live Demo:
See the Pen javascript-basic-exercise-69-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program that accepts a converging function and a list of branching functions and returns a function that applies each branching function to the arguments and the results of the branching functions are passed as arguments to the converging function.
Next: Write a JavaScript program to count the occurrences of a value in an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics