JavaScript: Perform left-to-right function composition
JavaScript fundamental (ES6 Syntax): Exercise-67 with Solution
Write a JavaScript program to perform left-to-right function composition.
- Use Array.prototype.reduce() to perform left-to-right function composition.
- The first (leftmost) function can accept one or more arguments; the remaining functions must be unary.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'composeRight' that takes any number of functions as arguments and returns a new function.
const composeRight = (...fns) => fns.reduce((f, g) => (...args) => g(f(...args)));
// Define functions 'add' and 'square'.
const add = (x, y) => x + y;
const square = x => x * x;
// Create a new function 'addAndSquare' by composing 'add' and 'square' in the right-to-left order.
const addAndSquare = composeRight(add, square);
// Example usage:
console.log(addAndSquare(1, 2)); // Output 9
console.log(addAndSquare(3, 2)); // Output 25
Output:
9 25
Visual Presentation:
Flowchart:
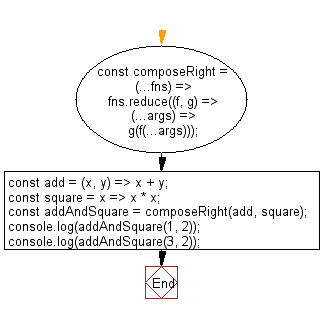
Live Demo:
See the Pen javascript-basic-exercise-67-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to perform right-to-left function composition.
Next: Write a JavaScript program that accepts a converging function and a list of branching functions and returns a function that applies each branching function to the arguments and the results of the branching functions are passed as arguments to the converging function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-67.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics