JavaScript: Add special characters to text to print in color in the console
JavaScript fundamental (ES6 Syntax): Exercise-65 with Solution
Add Console Log Colors
Write a JavaScript program to add special characters to text to print in color on the console (combined with console.log()).
- Use template literals and special characters to add the appropriate color code to the string output.
- For background colors, add a special character that resets the background color at the end of the string.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'colorize' that takes any number of arguments and returns an object with colorized versions of the input strings.
const colorize = (...args) => ({
black: `\x1b[30m${args.join(' ')}`, // Black color
red: `\x1b[31m${args.join(' ')}`, // Red color
green: `\x1b[32m${args.join(' ')}`, // Green color
yellow: `\x1b[33m${args.join(' ')}`, // Yellow color
blue: `\x1b[34m${args.join(' ')}`, // Blue color
magenta: `\x1b[35m${args.join(' ')}`, // Magenta color
cyan: `\x1b[36m${args.join(' ')}`, // Cyan color
white: `\x1b[37m${args.join(' ')}`, // White color
bgBlack: `\x1b[40m${args.join(' ')}\x1b[0m`, // Black background
bgRed: `\x1b[41m${args.join(' ')}\x1b[0m`, // Red background
bgGreen: `\x1b[42m${args.join(' ')}\x1b[0m`, // Green background
bgYellow: `\x1b[43m${args.join(' ')}\x1b[0m`, // Yellow background
bgBlue: `\x1b[44m${args.join(' ')}\x1b[0m`, // Blue background
bgMagenta: `\x1b[45m${args.join(' ')}\x1b[0m`, // Magenta background
bgCyan: `\x1b[46m${args.join(' ')}\x1b[0m`, // Cyan background
bgWhite: `\x1b[47m${args.join(' ')}\x1b[0m` // White background
});
// Example usage:
console.log(colorize('foo').red);
console.log(colorize('foo', 'bar').bgBlue);
console.log(colorize(colorize('foo').yellow, colorize('foo').green).bgWhite);
Output:
[31mfoo [44mfoo bar[0m [47m[33mfoo [32mfoo[0m
Flowchart:
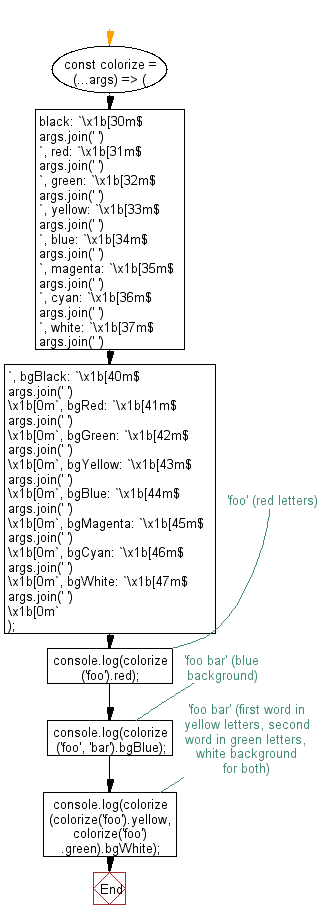
Live Demo:
See the Pen javascript-basic-exercise-65-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that prints console messages with custom colors using ANSI escape codes.
- Write a JavaScript function that wraps console.log to allow colorized output based on input parameters.
- Write a JavaScript program that logs different messages in different colors to distinguish log levels in the console.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get the first non-null / undefined argument.
Next: Write a JavaScript program to perform right-to-left function composition.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.