JavaScript: Chain asynchronous functions
JavaScript fundamental (ES6 Syntax): Exercise-62 with Solution
Chain Asynchronous Functions
Write a JavaScript program to chain asynchronous functions.
Note: Loop through an array of functions containing asynchronous events, calling next when each asynchronous event has completed.
- Loop through an array of functions containing asynchronous events, calling next when each asynchronous event has completed.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'chainAsync' to execute an array of asynchronous functions sequentially.
const chainAsync = fns => {
let curr = 0;
// Define a helper function 'next' to execute the next function in the array.
const next = () => fns[curr++](next);
// Start the chain by calling 'next'.
next();
};
// Call 'chainAsync' with an array of asynchronous functions.
chainAsync([
next => {
console.log('0 seconds');
setTimeout(next, 1000);
},
next => {
console.log('1 second');
}
]);
Output:
0 seconds 1 second
Flowchart:
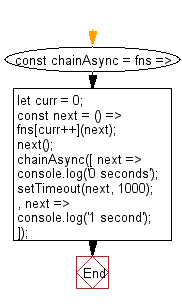
Live Demo:
See the Pen javascript-basic-exercise-62-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that executes an array of asynchronous functions sequentially using promises.
- Write a JavaScript function that chains asynchronous operations with async/await in a loop.
- Write a JavaScript program that manages a series of asynchronous tasks, ensuring each begins only after the previous one completes.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to cast the provided value as an array if it's not one.
Next: Write a JavaScript program to clone a given regular expression.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics