JavaScript: Create a function that invokes the method at a given key of an object
JavaScript fundamental (ES6 Syntax): Exercise-60 with Solution
Write a JavaScript program to create a function that invokes the method at a given key of an object. Optionally, add any parameters that are supplied to the beginning of the arguments.
- Return a function that uses Function.prototype.apply() to bind context[fn] to context.
- Use the spread operator (...) to prepend any additional supplied parameters to the arguments.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'bindKey' to bind a method of an object to that object.
const bindKey = (context, fn, ...args) =>
// Return a new function that applies the specified method of the context with the provided arguments.
function() {
return context[fn].apply(context, args.concat(...arguments));
};
// Define an object 'freddy' with a 'user' property and a 'greet' method.
const freddy = {
user: 'fred',
greet: function(greeting, punctuation) {
return greeting + ' ' + this.user + punctuation;
}
};
// Bind the 'greet' method of the 'freddy' object.
const freddyBound = bindKey(freddy, 'greet');
// Call the bound method with additional arguments.
console.log(freddyBound('hi', '!')); // Output: "hi fred!"
Output:
hi fred!
Flowchart:
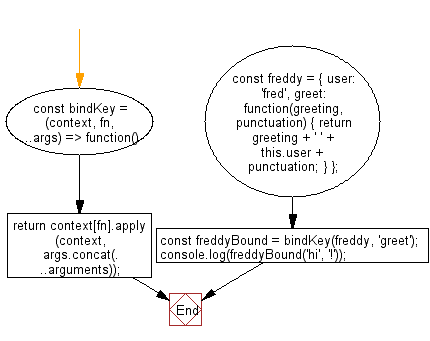
Live Demo:
See the Pen javascript-basic-exercise-60-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to create a function that invokes fn with a given context, optionally adding any additional supplied parameters to the beginning of the arguments.
Next: Write a JavaScript program to cast the provided value as an array if it's not one.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-60.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics