JavaScript: Target a given value in a nested JSON object
JavaScript fundamental (ES6 Syntax): Exercise-6 with Solution
Target Value in Nested JSON
Write a JavaScript program to target a given value in a nested JSON object based on the given key.
- Use the in operator to check if target exists in obj.
- If found, return the value of obj[target].
- Otherwise use Object.values(obj) and Array.prototype.reduce() to recursively call dig on each nested object until the first matching key/value pair is found.
Sample Solution:
JavaScript Code:
// Source: https://bit.ly/2neWfJ2
// Define a function called `dig` that searches for a target key in a nested object.
const dig = (obj, target) =>
target in obj // Check if the target key exists in the object.
? obj[target] // If found, return its value.
: Object.values(obj).reduce((acc, val) => { // If not found, recursively search in nested objects.
if (acc !== undefined) return acc; // If the target is already found, return it.
if (typeof val === 'object') return dig(val, target); // If the current value is an object, recursively search within it.
}, undefined); // Default return value if the target is not found.
// Test cases
const data = {
level1: {
level2: {
level3: 'some data'
}
}
};
const dog = {
"status": "success",
"message": "https://images.dog.ceo/breeds/african/n02116738_1105.jpg"
};
console.log(dig(data, 'level3')); // Output: 'some data'
console.log(dig(data, 'level4')); // Output: undefined
console.log(dig(dog, 'message')); // Output: 'https://images.dog.ceo/breeds/african/n02116738_1105.jpg'
Output:
some data undefined https://images.dog.ceo/breeds/african/n02116738_1105.jpg
Flowchart:
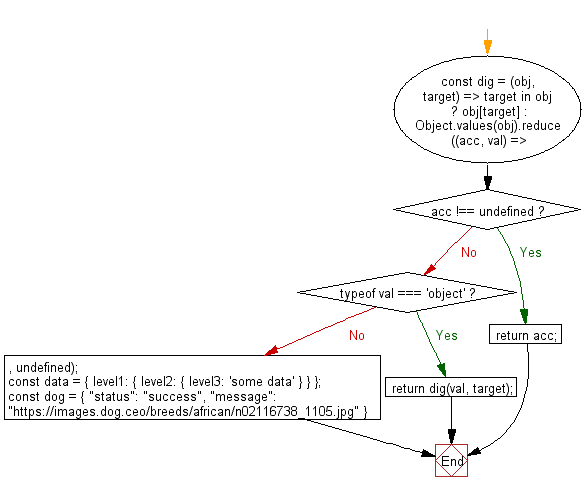
Live Demo:
See the Pen javascript-basic-exercise-1-6 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that recursively searches a nested JSON object for a given key and returns its associated value.
- Write a JavaScript function that traverses a complex JSON object to locate and return a specified target value.
- Write a JavaScript program that extracts all occurrences of a given key from a nested JSON object into an array.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to convert an array of objects to a comma-separated values (CSV) string that contains only the columns specified.
Next: Write a JavaScript program to converts a specified number to an array of digits.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.