JavaScript: Create a function that invokes fn with a given context
JavaScript fundamental (ES6 Syntax): Exercise-59 with Solution
Invoke Function in Given Context
Write a JavaScript program to create a function that invokes fn in a given context. Optionally add any additional variables to the arguments beginning.
- Return a function that uses Function.prototype.apply() to apply the given context to fn.
- Use the spread operator (...) to prepend any additional supplied parameters to the arguments.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'bind' to bind a context and arguments to a function.
const bind = (fn, context, ...args) =>
// Return a new function that applies the original function with the provided context and arguments.
function() {
return fn.apply(context, args.concat(...arguments));
};
// Define a function 'greet' to concatenate a greeting with a user and punctuation.
function greet(greeting, punctuation) {
return greeting + ' ' + this.user + punctuation;
}
// Define an object 'freddy' with a 'user' property.
const freddy = { user: 'Morning' };
// Bind the 'greet' function to the 'freddy' object.
const freddyBound = bind(greet, freddy);
// Call the bound function with additional arguments.
console.log(freddyBound('Good', '!')); // Output: "Good Morning!"
Output:
Good Morning!
Flowchart:
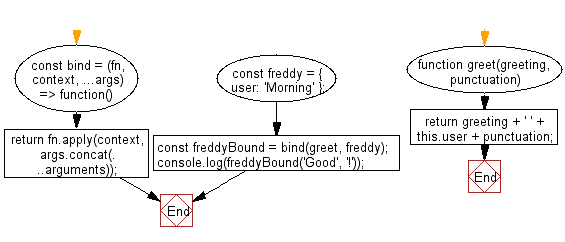
Live Demo:
See the Pen javascript-basic-exercise-59-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that creates a function which invokes another function with a specified this context.
- Write a JavaScript function that uses Function.prototype.call to execute a function in a given context.
- Write a JavaScript program that binds a function to an object and then immediately invokes it with additional arguments.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to split values into two groups according to a predicate function, which specifies which group an element in the input collection belongs to.
Next: Write a JavaScript program to create a function that invokes the method at a given key of an object, optionally adding any additional supplied parameters to the beginning of the arguments.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.