JavaScript: Split values into two groups according to a predicate function
JavaScript fundamental (ES6 Syntax): Exercise-58 with Solution
Split Values into Groups by Predicate
Write a JavaScript program to split values into two groups according to a predicate function. This specifies which group an element in the input collection belongs to.
If the predicate function returns a truthy value, the collection element belongs to the first group; otherwise, it belongs to the second group.
- Use Array.prototype.reduce() and Array.prototype.push() to add elements to groups, based on the value returned by fn for each element.
- If fn returns a truthy value for any element, add it to the first group, otherwise add it to the second group.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'bifurcateBy' to split an array into two groups based on the result of a function.
const bifurcateBy = (arr, fn) =>
// Reduce the array into two groups based on the truthiness of the function's result.
arr.reduce((acc, val, i) => (acc[fn(val, i) ? 0 : 1].push(val), acc), [[], []]);
// Test case
console.log(bifurcateBy(['beep', 'boop', 'foo', 'bar'], x => x[0] === 'b'));
// Output: [['beep', 'boop', 'bar'], ['foo']] (words starting with 'b' in the first group, others in the second group)
Output:
[["beep","boop","bar"],["foo"]]
Flowchart:
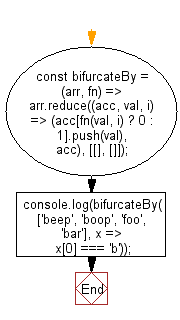
Live Demo:
See the Pen javascript-basic-exercise-58-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that splits an array into two groups based on a predicate function.
- Write a JavaScript function that returns an object with two arrays: one for elements passing the predicate and one for those that fail.
- Write a JavaScript program that partitions an array by evaluating each element with a boolean function.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to compute the average of an array, after mapping each element to a value using the provided function.
Next: Write a JavaScript program to create a function that invokes fn with a given context, optionally adding any additional supplied parameters to the beginning of the arguments.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics