JavaScript: Join all given URL segments together, then normalizes the resulting URL
JavaScript fundamental (ES6 Syntax): Exercise-55 with Solution
Join and Normalize URL Segments
Write a JavaScript program to join all given URL segments together, then normalize the resulting URL.
- Use String.prototype.join('/') to combine URL segments.
- Use a series of String.prototype.replace() calls with various regexps to normalize the resulting URL (remove double slashes, add proper slashes for protocol, remove slashes before parameters, combine parameters with '&' and normalize first parameter delimiter).
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'URL_Join' to join URL parts and handle various cases.
const URL_Join = (...args) =>
// Join the URL parts with a forward slash, then perform several replacements to handle different URL formatting cases.
args
.join('/')
.replace(/[\/]+/g, '/') // Replace multiple slashes with a single slash.
.replace(/^(.+):\//, '$1://') // Add a colon after the scheme if missing.
.replace(/^file:/, 'file:/') // Add a colon after 'file' if missing.
.replace(/\/(\?|&|#[^!])/g, '$1') // Remove slashes before query parameters or fragments.
.replace(/\?/g, '&') // Replace the first occurrence of '?' with '&'.
.replace('&', '?'); // If there are multiple query parameters, replace the first '&' with '?'.
// Test case
console.log(URL_Join('http://www.google.com', 'a', '/b/cd', '?foo=123', '?bar=foo')); // Output: http://www.google.com/a/b/cd?foo=123&bar=foo
Output:
http://www.google.com/a/b/cd?foo=123&bar=foo
Visual Presentation:
Flowchart:
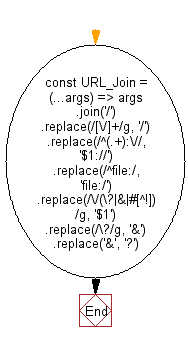
Live Demo:
See the Pen javascript-basic-exercise-55-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that joins multiple URL segments into a normalized URL, removing duplicate slashes.
- Write a JavaScript function that concatenates URL parts and ensures proper formatting using regex replacements.
- Write a JavaScript program that takes an array of URL fragments and constructs a well-formed URL path.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to initialize an array containing the numbers in the specified range where start and end are inclusive with their common difference step.
Next: Write a JavaScript program to check if all elements in a given array are equal or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.