JavaScript: Initialize an array containing the numbers in the specified range where start and end are inclusive with their common difference step
JavaScript fundamental (ES6 Syntax): Exercise-54 with Solution
Array of Numbers in Specified Range
Write a JavaScript program to initialize an array containing numbers in the specified range. Start and end are inclusive of their common point of difference.
- Use Array.from() to create an array of the desired length.
- Use (end - start + 1)/step and a map function to fill the array with the desired values in the given range.
- Omit the second argument, start, to use a default value of 0.
- Omit the last argument, step, to use a default value of 1.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'initialize_Array_With_Range' to create an array filled with a range of numbers.
const initialize_Array_With_Range = (end, start = 0, step = 1) =>
// Create an array with the length calculated based on the range and map each index to its corresponding value based on the step.
Array.from({ length: Math.ceil((end + 1 - start) / step) }).map((v, i) => i * step + start);
// Test cases
console.log(initialize_Array_With_Range(5)); // Output: [ 0, 1, 2, 3, 4, 5 ]
console.log(initialize_Array_With_Range(8, 3)); // Output: [ 3, 4, 5, 6, 7, 8 ]
console.log(initialize_Array_With_Range(6, 0, 2)); // Output: [ 0, 2, 4, 6 ]
Output:
[0,1,2,3,4,5] [3,4,5,6,7,8] [0,2,4,6]
Visual Presentation:
Flowchart:
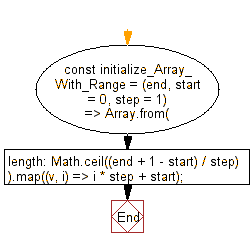
Live Demo:
See the Pen javascript-basic-exercise-1-54 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that creates an array of consecutive numbers within a specified inclusive range.
- Write a JavaScript function that generates an array from a start number to an end number using Array.from().
- Write a JavaScript program that validates range inputs and returns an array containing each number in that interval.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to Initialize a two dimension array of given width and height and value.
Next: Write a JavaScript program to Join all given URL segments together, then normalizes the resulting URL.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.