JavaScript: Initialize a two dimension array of given width and height and value
JavaScript fundamental (ES6 Syntax): Exercise-53 with Solution
Initialize 2D Array with Size and Value
Write a JavaScript program to initialize a two-dimensional array of given size and value.
- Use Array.from() and Array.prototype.map() to generate h rows where each is a new array of size w.
- Use Array.prototype.fill() to initialize all items with value val.
- Omit the last argument, val, to use a default value of null.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'initialize_2D_Array' to create a 2D array with a specified width, height, and default value.
const initialize_2D_Array = (w, h, val = null) =>
// Create an array with the specified height and map each element to an array with the specified width filled with the default value.
Array.from({ length: h }).map(() => Array.from({ length: w }).fill(val));
// Test cases
console.log(initialize_2D_Array(2, 2, 0)); // Output: [ [ 0, 0 ], [ 0, 0 ] ]
console.log(initialize_2D_Array(3, 3, 3)); // Output: [ [ 3, 3, 3 ], [ 3, 3, 3 ], [ 3, 3, 3 ] ]
Output:
[[0,0],[0,0]] [[3,3,3],[3,3,3],[3,3,3]]
Visual Presentation:
Flowchart:
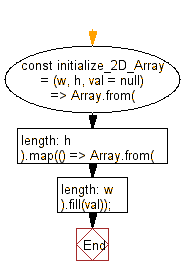
Live Demo:
See the Pen javascript-basic-exercise-1-53 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that creates a two-dimensional array of given dimensions, filled with a specified value.
- Write a JavaScript function that initializes an m x n matrix with a default value using nested loops.
- Write a JavaScript program that generates a 2D array with dynamic size and populates each element with a function result.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to group the elements of an given array based on the given function.
Next: Write a JavaScript program to initialize an array containing the numbers in the specified range where start and end are inclusive with their common difference step.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.