JavaScript: Group the elements of a given array based on the given function
JavaScript fundamental (ES6 Syntax): Exercise-52 with Solution
Group Array Elements by Function
Write a JavaScript program to group the elements of a given array based on the given function.
- Use Array.prototype.map() to map the values of the array to a function or property name.
- Use Array.prototype.reduce() to create an object, where the keys are produced from the mapped results.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'group_By' to group elements of an array based on a specified function.
const group_By = (arr, fn) =>
// Map the array elements to keys using the provided function, then reduce into groups based on keys.
arr.map(typeof fn === 'function' ? fn : val => val[fn]).reduce((acc, val, i) => {
acc[val] = (acc[val] || []).concat(arr[i]); // Create groups based on keys.
return acc;
}, {});
// Test cases
console.log(group_By([6.1, 4.2, 6.3], Math.sqrt)); // Output: { '2.463751439371663': [ 6.1, 6.3 ], '2.04939015319192': [ 4.2 ] }
console.log(group_By([6.1, 4.2, 6.3], Math.floor)); // Output: { '6': [ 6.1, 6.3 ], '4': [ 4.2 ] }
console.log(group_By(['one', 'two', 'three'], 'length')); // Output: { '3': [ 'one', 'two' ], '5': [ 'three' ] }
Output:
{"2.4698178070456938":[6.1],"2.04939015319192":[4.2],"2.5099800796022267":[6.3]} {"4":[4.2],"6":[6.1,6.3]} {"3":["one","two"],"5":["three"]}
Flowchart:
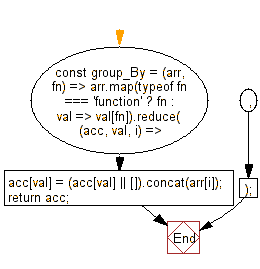
Live Demo:
See the Pen javascript-basic-exercise-1-52 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that groups elements of an array based on the output of a provided function.
- Write a JavaScript function that organizes array items into buckets where each bucket corresponds to a computed key.
- Write a JavaScript program that returns an object where keys are the results of the grouping function and values are arrays of elements.
Go to:
PREV : Get Current URL Parameters as Object.
NEXT : Initialize 2D Array with Size and Value.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.