JavaScript: Get an object containing the parameters of the current URL
JavaScript fundamental (ES6 Syntax): Exercise-51 with Solution
Get Current URL Parameters as Object
Write a JavaScript program to get an object containing the current URL parameters.
- Use String.prototype.match() with an appropriate regular expression to get all key-value pairs.
- Use Array.prototype.reduce() to map and combine them into a single object.
- Pass location.search as the argument to apply to the current url.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'get_URL_Parameters' to extract URL parameters from a given URL.
const get_URL_Parameters = url =>
// Use regex to match parameter-value pairs in the URL, then reduce the matches into an object.
(url.match(/([^?=&]+)(=([^&]*))/g) || []).reduce(
(a, v) => ((a[v.slice(0, v.indexOf('='))] = v.slice(v.indexOf('=') + 1)), a),
{}
);
// Test cases
console.log(get_URL_Parameters('http://url.com/page?name=Adam&surname=Smith')); // Output: { name: 'Adam', surname: 'Smith' }
console.log(get_URL_Parameters('google.com')); // Output: {}
console.log(get_URL_Parameters('https://www.w3resource.com')); // Output: {}
Output:
{"name":"Adam","surname":"Smith"} {} {}
Flowchart:
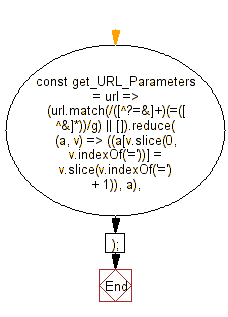
Live Demo:
See the Pen javascript-basic-exercise-1-51 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that parses the current page URL and returns an object containing its query parameters.
- Write a JavaScript function that converts a URL's search string into a key-value object using URLSearchParams.
- Write a JavaScript program that extracts URL parameters and handles cases with duplicate keys by storing values in an array.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to convert an integer to a suffixed string, adding am or pm based on its value.
Next: Write a JavaScript program to group the elements of an given array based on the given function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics