JavaScript : Convert an integer to a suffixed string, adding am or pm based on its value
JavaScript fundamental (ES6 Syntax): Exercise-50 with Solution
Integer to Suffix String with am/pm
Write a JavaScript program to convert an integer to a suffixed string, adding am or pm based on its value.
- Use the modulo operator (%) and conditional checks to transform an integer to a stringified 12-hour format with meridiem suffix.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'get_Meridiem_Suffix_Of_Integer' that returns the meridiem suffix ('am' or 'pm') of an integer.
const get_Meridiem_Suffix_Of_Integer = num =>
// Check various cases and return the appropriate suffix.
num === 0 || num === 24
? 12 + 'am'
: num === 12
? 12 + 'pm'
: num < 12
? (num % 12) + 'am'
: (num % 12) + 'pm';
// Test cases
console.log(get_Meridiem_Suffix_Of_Integer(0)); // Output: '12am'
console.log(get_Meridiem_Suffix_Of_Integer(11)); // Output: '11am'
console.log(get_Meridiem_Suffix_Of_Integer(13)); // Output: '1pm'
console.log(get_Meridiem_Suffix_Of_Integer(25)); // Output: '1pm'
Output:
12am 11am 1pm 1pm
Visual Presentation:
Flowchart:
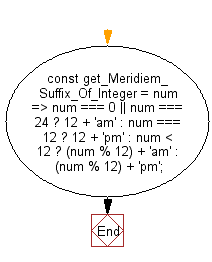
Live Demo:
See the Pen javascript-basic-exercise-1-49 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that converts an integer hour to a string with an "am" or "pm" suffix based on its value.
- Write a JavaScript function that formats a 24-hour clock number into a 12-hour clock string with the appropriate suffix.
- Write a JavaScript program that validates an integer input and returns its time period designation as "am" or "pm".
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to retrieve a set of properties indicated by the given selectors from an object.
Next: Write a JavaScript program to get an object containing the parameters of the current URL.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics