JavaScript: Convert an array of objects to a comma-separated values string that contains only the columns specified
JavaScript fundamental (ES6 Syntax): Exercise-5 with Solution
Array of Objects to CSV String
Write a JavaScript program to convert an array of objects to a comma-separated value (CSV) string that contains only the columns specified.
- Use Array.prototype.join(delimiter) to combine all the names in columns to create the first row.
- Use Array.prototype.map() and Array.prototype.reduce() to create a row for each object, substituting non-existent values with empty strings and only mapping values in columns.
- Use Array.prototype.join('\n') to combine all rows into a string.
- Omit the third argument, delimiter, to use a default delimiter of,.
Sample Solution:
JavaScript Code:
// Source: https://bit.ly/2neWfJ2
// Define a function called `JSON_to_CSV` that converts JSON data to CSV format.
const JSON_to_CSV = (arr, columns, delimiter = ',') =>
[
// Combine column titles with the delimiter.
columns.join(delimiter),
// Map each object in the array to a CSV row.
...arr.map(obj =>
// For each object, reduce the values corresponding to the specified columns into a CSV row.
columns.reduce(
(acc, key) => `${acc}${!acc.length ? '' : delimiter}"${!obj[key] ? '' : obj[key]}"`, // Format value within double quotes.
''
)
)
].join('\n'); // Join rows with newline characters.
// Test cases
console.log(JSON_to_CSV([{ x: 100, y: 200 }, { x: 300, y: 400, z: 500 }, { x: 6 }, { y: 7 }], ['x', 'y']));
console.log(JSON_to_CSV([{ x: 100, y: 200 }, { x: 300, y: 400, z: 500 }, { x: 6 }, { y: 7 }], ['x', 'y'], ';'));
Sample Output:
x,y "100","200" "300","400" "6","" "","7" x;y "100";"200" "300";"400" "6";"" "";"7"
Flowchart:
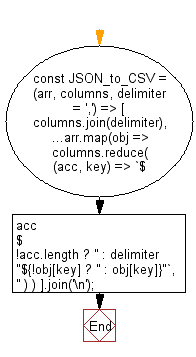
Live Demo:
See the Pen javascript-basic-exercise-1-5 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that converts an array of objects to a CSV string including only specified columns.
- Write a JavaScript function that serializes an array of objects into CSV format, ensuring proper quoting for special characters.
- Write a JavaScript program that maps object keys to CSV headers and concatenates the values accordingly.
Go to:
PREV : CSV to 2D Array of Objects.
NEXT : Target Value in Nested JSON.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.