JavaScript: Retrieve a set of properties indicated by the given selectors from an object
JavaScript fundamental (ES6 Syntax): Exercise-49 with Solution
Retrieve Selected Properties from Object
Write a JavaScript program to retrieve a set of properties indicated by the given selectors from an object.
- Use Array.prototype.map() for each selector, String.prototype.replace() to replace square brackets with dots.
- Use String.prototype.split('.') to split each selector.
- Use Array.prototype.filter() to remove empty values and Array.prototype.reduce() to get the value indicated by each selector.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'get' that retrieves values from an object based on selector strings.
const get = (from, ...selectors) =>
// Map over the selectors array.
[...selectors].map(s =>
// Replace square brackets with dots, split by dots, filter out empty strings,
// and then recursively retrieve the value from the object.
s
.replace(/\[([^\[\]]*)\]/g, '.$1.')
.split('.')
.filter(t => t !== '')
.reduce((prev, cur) => prev && prev[cur], from)
);
// Define an object 'obj' with nested properties.
const obj = { selector: { to: { val: 'val to select' } }, target: [1, 2, { a: 'test' }] };
// Use the 'get' function to retrieve values from 'obj' based on selectors.
console.log(get(obj, 'selector.to.val', 'target[0]', 'target[2].a'));
// Output: [ 'val to select', 1, 'test' ]
Output:
["val to select",1,"test"]
Flowchart:
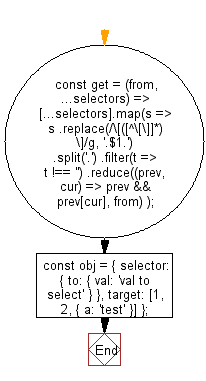
Live Demo:
See the Pen javascript-basic-exercise-1-49 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that returns a new object containing only the specified keys from the original object.
- Write a JavaScript function that uses array filtering to extract selected properties from an object based on a key list.
- Write a JavaScript program that destructures an object to retrieve only the desired properties and reassembles them into a new object.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get an array of function property names from own (and optionally inherited) enumerable properties of an object.
Next: Write a JavaScript program to convert an integer to a suffixed string, adding am or pm based on its value.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics