JavaScript: Get an array of function property names from own enumerable properties of an object
JavaScript fundamental (ES6 Syntax): Exercise-48 with Solution
Array of Function Property Names
Write a JavaScript program to get an array of function property names from the own (and optionally inherited) enumerable properties of an object.
- Use Object.keys(obj) to iterate over the object's own properties.
- If inherited is true, use Object.getPrototypeOf(obj) to also get the object's inherited properties.
- Use Array.prototype.filter() to keep only those properties that are functions.
- Omit the second argument, inherited, to not include inherited properties by default.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'functions' that retrieves the names of all functions in an object.
const functions = (obj, inherited = false) =>
// Check if 'inherited' is true.
(inherited
? // If true, concatenate the keys of the object and its prototype.
[...Object.keys(obj), ...Object.keys(Object.getPrototypeOf(obj))]
: // If false, get only the keys of the object.
Object.keys(obj)
)
// Filter the keys to include only those with function values.
.filter(key => typeof obj[key] === 'function');
// Define a constructor function 'Foo'.
function Foo() {
// Assign arrow function expressions to properties 'a' and 'b'.
this.a = () => 1;
this.b = () => 2;
}
// Assign an arrow function expression to the prototype property 'c'.
Foo.prototype.c = () => 3;
// Create an instance of 'Foo' and log the functions in the instance.
console.log(functions(new Foo())); // Output: [ 'a', 'b' ]
// Create an instance of 'Foo' and include inherited functions, then log them.
console.log(functions(new Foo(), true)); // Output: [ 'a', 'b', 'c' ]
Output:
["a","b"] ["a","b","c"]
Flowchart:
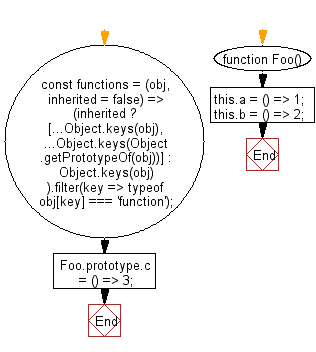
Live Demo:
See the Pen javascript-basic-exercise-1-47 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that extracts the names of all function properties from an object, including inherited ones.
- Write a JavaScript function that returns an array of keys from an object whose values are functions.
- Write a JavaScript program that filters an object’s properties to list only those that are callable.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to perform a deep comparison between two values to determine if they are equivalent.
Next: Write a JavaScript program to retrieve a set of properties indicated by the given selectors from an object.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.