JavaScript: Change function that accepts an array into a variadic function
JavaScript fundamental (ES6 Syntax): Exercise-43 with Solution
Change Function to Variadic
Write a JavaScript program to change a function that accepts an array into a variadic function.
Note: Given a function, return a closure that collects all inputs into an array-accepting function.
- Given a function, return a closure that collects all inputs into an array-accepting function.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'collectInto' that takes a function 'fn' as input and returns another function.
const collectInto = fn =>
// The returned function takes any number of arguments 'args'.
(...args) =>
// Call the original function 'fn' with 'args' collected into an array.
fn(args);
// Define a new function 'Pall' using 'collectInto' that collects all promises into a single promise.
const Pall = collectInto(Promise.all.bind(Promise));
// Create three promises 'p1', 'p2', and 'p3'.
let p1 = Promise.resolve(1);
let p2 = Promise.resolve(2);
let p3 = new Promise(resolve => setTimeout(resolve, 2000, 3));
// Call 'Pall' with the promises 'p1', 'p2', and 'p3' and log the result.
Pall(p1, p2, p3).then(console.log); // Output: [1, 2, 3] (after about 2 seconds)
Output:
[1,2,3]
Flowchart:
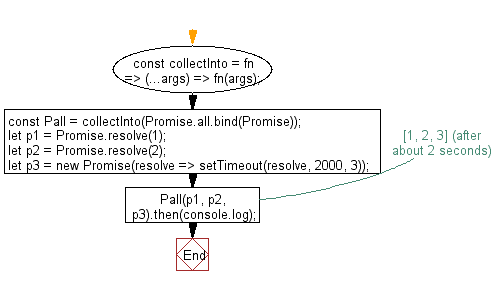
Live Demo:
See the Pen javascript-basic-exercise-1-43 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that converts a function expecting an array into a variadic function using the spread operator.
- Write a JavaScript function that wraps an existing function to allow it to accept multiple arguments instead of a single array.
- Write a JavaScript program that transforms a fixed-argument function into a variadic one while preserving its behavior.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get a customized coalesce function that returns the first argument that returns true from the provided argument validation function.
Next: Write a JavaScript program to remove falsey values from an given array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.