JavaScript: Create an object from the given key-value pairs
JavaScript fundamental (ES6 Syntax): Exercise-41 with Solution
Object from Key-Value Pairs
Write a JavaScript program to create an object from the given key-value pairs.
- Use Array.prototype.reduce() to create and combine key-value pairs.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'object_From_Pairs' that takes an array 'arr' of key-value pairs as input.
const object_From_Pairs = arr =>
// Reduce the array to an object by iteratively assigning key-value pairs.
arr.reduce((a, v) => ((a[v[0]] = v[1]), a), {});
// Test the 'object_From_Pairs' function with different arrays of key-value pairs and output the resulting objects.
console.log(object_From_Pairs([['a', 1], ['b', 2]])); // Output: { a: 1, b: 2 }
console.log(object_From_Pairs([[1, 10], [2, 20], [3, 30]])); // Output: { '1': 10, '2': 20, '3': 30 }
Output:
{"a":1,"b":2} {"1":10,"2":20,"3":30}
Visual Presentation:
Flowchart:
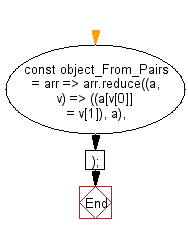
Live Demo:
See the Pen javascript-basic-exercise-1-41 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that creates an object from an array of key-value pair arrays.
- Write a JavaScript function that reconstructs an object using the reduce() method on an array of key-value pairs.
- Write a JavaScript program that merges an array of [key, value] pairs into an object, handling duplicate keys by overwriting.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to create an array of key-value pair arrays from an given object.
Next: Write a JavaScript program to get a customized coalesce function that returns the first argument that returns true from the provided argument validation function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.