JavaScript: Create an array of key-value pair arrays from a given object
JavaScript fundamental (ES6 Syntax): Exercise-40 with Solution
Write a JavaScript program to create an array of key-value pair arrays from a given object.
- Use Object.entries() to get an array of key-value pair arrays from the given object.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'object_to_pairs' that takes an object 'obj' as input.
const object_to_pairs = obj =>
// Map over the keys of the object and return an array of key-value pairs.
Object.keys(obj).map(k => [k, obj[k]]);
// Test the 'object_to_pairs' function with different objects and output the resulting arrays of key-value pairs.
console.log(object_to_pairs({ a: 1, b: 2 })); // Output: [['a', 1], ['b', 2]]
console.log(object_to_pairs({ a: 1, b: 2, c: 3 })); // Output: [['a', 1], ['b', 2], ['c', 3]]
Output:
[["a",1],["b",2]] [["a",1],["b",2],["c",3]]
Visual Presentation:
Flowchart:
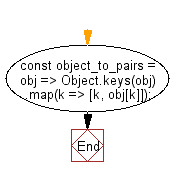
Live Demo:
See the Pen javascript-basic-exercise-1-40 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to remove the key-value pairs corresponding to the given keys from an object.
Next: Write a JavaScript program to create an object from the given key-value pairs.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-40.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics