JavaScript : Convert a comma-separated values string to a 2D array of objects
JavaScript fundamental (ES6 Syntax): Exercise-4 with Solution
CSV to 2D Array of Objects
Write a JavaScript program to convert a comma-separated value (CSV) string to a 2D array of objects. The first row of the string is used as the title row.
- Use Array.prototype.slice() and Array.prototype.indexOf('\n') and String.prototype.split(delimiter) to separate the first row (title row) into values.
- Use String.prototype.split('\n') to create a string for each row, then Array.prototype.map() and String.prototype.split(delimiter) to separate the values in each row.
- Use Array.prototype.reduce() to create an object for each row's values, with the keys parsed from the title row.
- Omit the second argument, delimiter, to use a default delimiter of ,.
Sample Solution:
JavaScript Code:
// Source: https://bit.ly/2neWfJ2
// Define a function called `CSV_to_JSON` that converts CSV data to a JSON array.
const CSV_to_JSON = (data, delimiter = ',') => {
// Extract titles from the first row of the CSV data.
const titles = data.slice(0, data.indexOf('\n')).split(delimiter);
// Split the CSV data by newline characters, map each row to a JSON object with titles as keys.
return data
.slice(data.indexOf('\n') + 1)
.split('\n')
.map(v => {
const values = v.split(delimiter);
// Create a JSON object using titles and corresponding values.
return titles.reduce((obj, title, index) => ((obj[title] = values[index]), obj), {});
});
};
// Test cases
console.log(CSV_to_JSON('col1,col2\na,b\nc,d')); // [{'col1': 'a', 'col2': 'b'}, {'col1': 'c', 'col2': 'd'}];
console.log(CSV_to_JSON('col1;col2\na;b\nc;d', ';')); // [{'col1': 'a', 'col2': 'b'}, {'col1': 'c', 'col2': 'd'}];
Output:
[{"col1":"a","col2":"b"},{"col1":"c","col2":"d"}] [{"col1":"a","col2":"b"},{"col1":"c","col2":"d"}]
Flowchart:
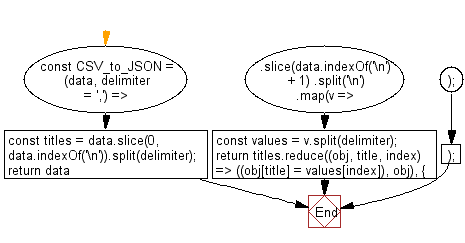
Live Demo:
See the Pen javascript-basic-exercise-1-4 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to converts a comma-separated values (CSV) string to a 2D array.
Next: Write a JavaScript program to convert an array of objects to a comma-separated values (CSV) string that contains only the columns specified.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics