JavaScript: Remove the key-value pairs corresponding to the given keys from an object
JavaScript fundamental (ES6 Syntax): Exercise-39 with Solution
Remove Keys from Object
Write a JavaScript program to remove the key-value pairs corresponding to the given keys from an object.
- Use Object.keys(), Array.prototype.filter() and Array.prototype.includes() to remove the provided keys.
- Use Array.prototype.reduce() to convert the filtered keys back to an object with the corresponding key-value pairs.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'omit' that takes two parameters: 'obj' (an object) and 'arr' (an array of keys to omit).
const omit = (obj, arr) =>
// Filter the keys of the object to exclude those included in the 'arr' array. Then, reduce the filtered keys to a new object.
Object.keys(obj)
.filter(k => !arr.includes(k))
.reduce((acc, key) => ((acc[key] = obj[key]), acc), {});
// Test the 'omit' function with different objects and arrays of keys to omit, then output the resulting objects.
console.log(omit({ a: 1, b: '2', c: 3 }, ['b'])); // Output: { a: 1, c: 3 }
console.log(omit({ a: 1, b: 2, c: 3 }, ['c'])); // Output: { a: 1, b: 2 }
Output:
{"a":1,"c":3} {"a":1,"b":2}
Visual Presentation:
Flowchart:
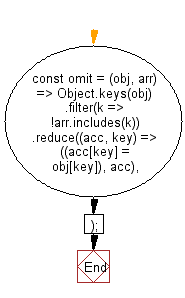
Live Demo:
See the Pen javascript-basic-exercise-1-39 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that removes specified key-value pairs from an object and returns a new object.
- Write a JavaScript function that deletes keys from an object based on an array of keys to remove.
- Write a JavaScript program that iterates over an object and omits keys that match a given blacklist.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to pad a string on both sides with the specified character, if it's shorter than the specified length.
Next: Write a JavaScript program to create an array of key-value pair arrays from an given object.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.