JavaScript: Pad a string on both sides with the specified character
JavaScript fundamental (ES6 Syntax): Exercise-38 with Solution
Pad String on Both Sides
Write a JavaScript program to pad a string on both sides with the specified character, if it's shorter than the specified length.
- Use String.prototype.padStart() and String.prototype.padEnd() to pad both sides of the given string.
- Omit the third argument, char, to use the whitespace character as the default padding character.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'pad' that takes three parameters: 'str' (a string), 'length' (the desired length of the padded string), and 'char' (the padding character, default is a space).
const pad = (str, length, char = ' ') =>
// Pad the string with the specified character using padStart and padEnd methods to achieve the desired length.
str.padStart((str.length + length) / 2, char).padEnd(length, char);
// Test the 'pad' function with different inputs and output the padded strings.
console.log(pad('cat', 8));
console.log(pad(String(42), 6, '0'));
console.log(pad('foobar', 3));
Output:
cat 004200 foobar
Visual Presentation:
Flowchart:
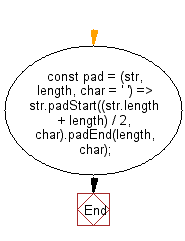
Live Demo:
See the Pen javascript-basic-exercise-1-38 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that pads a string on both sides with a specified character until it reaches a given length.
- Write a JavaScript function that centers a string by adding equal padding to the left and right using string.repeat().
- Write a JavaScript program that verifies the string’s current length and applies side padding if it is shorter than a target length.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get a sorted array of objects ordered by properties and orders.
Next: Write a JavaScript program to remove the key-value pairs corresponding to the given keys from an object.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics