JavaScript: Get an array of given n random integers in the specified range
JavaScript fundamental (ES6 Syntax): Exercise-35 with Solution
Array of n Random Integers in Range
Write a JavaScript program to get an array of given n random integers in the specified range.
- Use Array.from() to create an empty array of the specific length.
- Use Math.random() to generate random numbers and map them to the desired range, using Math.floor() to make them integers.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function named random_intArray_In_Range that generates an array of random integers within a specified range.
const random_intArray_In_Range = (min, max, n = 1) =>
Array.from({ length: n }, () => Math.floor(Math.random() * (max - min + 1)) + min);
// Test the function with different range values and output the results.
console.log(random_intArray_In_Range(1, 20, 10)); // Output: Array of 10 random integers between 1 and 20
console.log(random_intArray_In_Range(-10, 10, 5)); // Output: Array of 5 random integers between -10 and 10
Output:
[10,5,17,4,19,5,4,6,9,2] [7,-6,2,-9,-2]
Visual Presentation:
Flowchart:
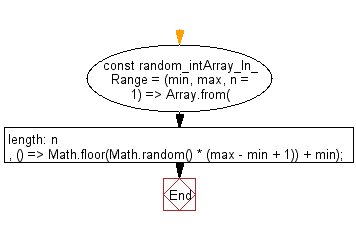
Live Demo:
See the Pen javascript-basic-exercise-35-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that generates an array of n random integers within a specified range.
- Write a JavaScript function that creates an array by repeatedly calling a random integer generator for a given count.
- Write a JavaScript program that validates n and the range values before populating an array with random integers.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get a random integer in the specified range.
Next: Write a JavaScript program to create a function that invokes each provided function with the arguments it receives and returns the results.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics