JavaScript: Get a random integer in the specified range
JavaScript fundamental (ES6 Syntax): Exercise-34 with Solution
Random Integer in Range
Write a JavaScript program to generate a random integer in the specified range.
- Use Math.random() to generate a random number and map it to the desired range.
- Use Math.floor() to make it an integer.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function named randomIntegerInRange that generates a random integer within a specified range.
const randomIntegerInRange = (min, max) => Math.floor(Math.random() * (max - min + 1)) + min;
// Test the function with different range values and output the results.
console.log(randomIntegerInRange(0, 5)); // Output: Random integer between 0 and 5
console.log(randomIntegerInRange(2, 5)); // Output: Random integer between 2 and 5
console.log(randomIntegerInRange(5, -5)); // Output: Random integer between 5 and -5
console.log(randomIntegerInRange(-2, -7)); // Output: Random integer between -2 and -7
Output:
1 3 -1 -4
Visual Presentation:
Flowchart:
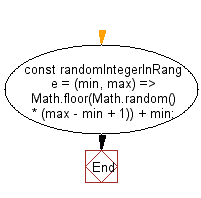
Live Demo:
See the Pen javascript-basic-exercise-1-34 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that generates a random integer within a specified range using Math.floor and Math.random.
- Write a JavaScript function that takes two integers as bounds and returns a random integer between them, inclusive.
- Write a JavaScript program that checks for valid integer bounds before returning a random integer in that range.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get a random number in the specified range.
Next: Write a JavaScript program to get an array of given n random integers in the specified range.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.