JavaScript: Get a random number in the specified range
JavaScript fundamental (ES6 Syntax): Exercise-33 with Solution
Random Number in Range
Write a JavaScript program to generate a random number in the specified range.
- Use Math.random() to generate a random value, map it to the desired range using multiplication.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function named random_Number_In_Range that generates a random number within a specified range.
const random_Number_In_Range = (min, max) => Math.random() * (max - min) + min;
// Test the function with different range values and output the results.
console.log(random_Number_In_Range(2, 10)); // Output: Random number between 2 and 10
console.log(random_Number_In_Range(1, 5)); // Output: Random number between 1 and 5
console.log(random_Number_In_Range(-5, -2)); // Output: Random number between -5 and -2
console.log(random_Number_In_Range(0, 1)); // Output: Random number between 0 and 1
Output:
5.897778190464821 1.1596294444724986 -2.644812110240778 0.5654096114937668
Visual Presentation:
Flowchart:
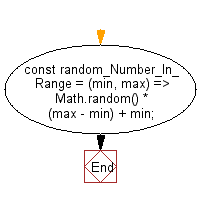
Live Demo:
See the Pen javascript-basic-exercise-1-33 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that generates a random floating-point number within a specified range using Math.random().
- Write a JavaScript function that returns a random number between two given bounds, inclusive of the lower bound.
- Write a JavaScript program that validates the range inputs and then produces a random number within that interval.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get the sum of an given array, after mapping each element to a value using the provided function.
Next: Write a JavaScript program to get a random integer in the specified range.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.